1.Java调用shell
Java语言以其跨平台性和简易性而著称,在Java里面的lang包里(java.lang.Runtime)提供了一个允许Java程序与该程序所运行的环境交互的接口,这就是Runtime类,在Runtime类里提供了获取当前运行环境的接口。
其中的exec函数返回一个执行shell命令的子进程。exec函数的具体实现形式有以下几种:
public Process exec(String command) throws IOException
public Process exec(String command,String[] envp) throws IOException
public Process exec(String command,String[] envp,File dir) throws IOException
public Process exec(String[] cmdarray) throws IOException
public Process exec(String[] cmdarray, String[] envp) throws IOException
public Process exec(String[] cmdarray, String[] envp,File dir) throws IOException
我们在这里主要用到的是第一个和第四个函数,具体方法很简单,就是在exec函数中传递一个代表命令的字符串。exec函数返回的是一个Process类型的类的实例。Process类主要用来控制进程,获取进程信息等作用。(具体信息及其用法请参看Java doc)。
1)执行简单的命令的方法:
代码如下:
try{
String commands = "ls -l";
Process process = Runtime.getRuntime().exec (commands);
// for showing the info on screen
InputStreamReader ir=new InputStreamReader(process.getInputStream());
BufferedReader input = new BufferedReader (ir);
String line;
while ((line = input.readLine ()) != null){
System.out.println(line);
}//end try
}
catch (java.io.IOException e){
System.err.println ("IOException " + e.getMessage());
}
上面的代码首先是声明了一个代表命令的字符串commands,它代表了ls -l 这个命令。之后我们用Runtime.getRuntime().exec(commands)来生成一个子进程来执行这个命令,如果这句话运行成功,则
命令 ls -l 运行成功(由于没有让它显示,不会显示ls -l的结果)。后面的流操作则是获取进程的流信息,并把它们一行行输出到屏幕。
2)执行带有参数的命令(尤其是参数需要用引号的)时则需要用String的数组来表示整个命令,而且要用转义符把引号的特殊含义去除,例如我们要执行
find / -name "*mysql*" -print 时,用如下代码
try {
String[] commands = new String[]{"find",".","-name","*mysql*","-print"};
Process process = Runtime.getRuntime().exec (commands);
InputStreamReader ir=new InputStreamReader(process.getInputStream());
BufferedReader input = new BufferedReader (ir);
String line;
while ((line = input.readLine ()) != null){
System.out.println(line);
}//end try
}
catch (java.io.IOException e){
System.err.println ("IOException " + e.getMessage());
}
Java 可以通过 Runtime 调用Linux命令,形式如下:
Runtime.getRuntime().exec(command)
但是这样执行时没有任何输出,因为调用 Runtime.exec 方法将产生一个本地的进程,并返回一个Process子类的实例(注意:Runtime.getRuntime().exec(command)返回的是一个Process类的实例)该实例可用于控制进程或取得进程的相关信息。
由于调用 Runtime.exec 方法所创建的子进程没有自己的终端或控制台,因此该子进程的标准IO(如stdin,stdou,stderr)都通过 Process.getOutputStream(),Process.getInputStream(), Process.getErrorStream() 方法重定向给它的父进程了。
用户需要用这些stream来向子进程输入数据或获取子进程的输出,下面的代码可以取到 linux 命令的执行结果:
try {
String[] cmd = new String[]{”/bin/sh”, “-c”, ” ls “};
Process ps = Runtime.getRuntime().exec(cmd);
BufferedReader br = new BufferedReader(new InputStreamReader(ps.getInputStream()));
StringBuffer sb = new StringBuffer();
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append(”\n”);
}
String result = sb.toString();
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
(文/boy)
文章来源:http://wangbaoaiboy.blog.163.com/blog/static/52111910201111892938552/
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
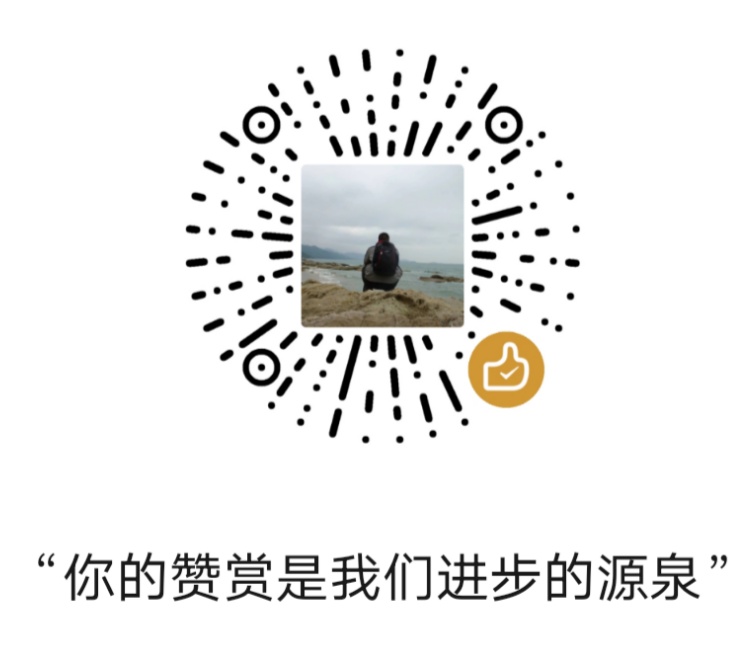
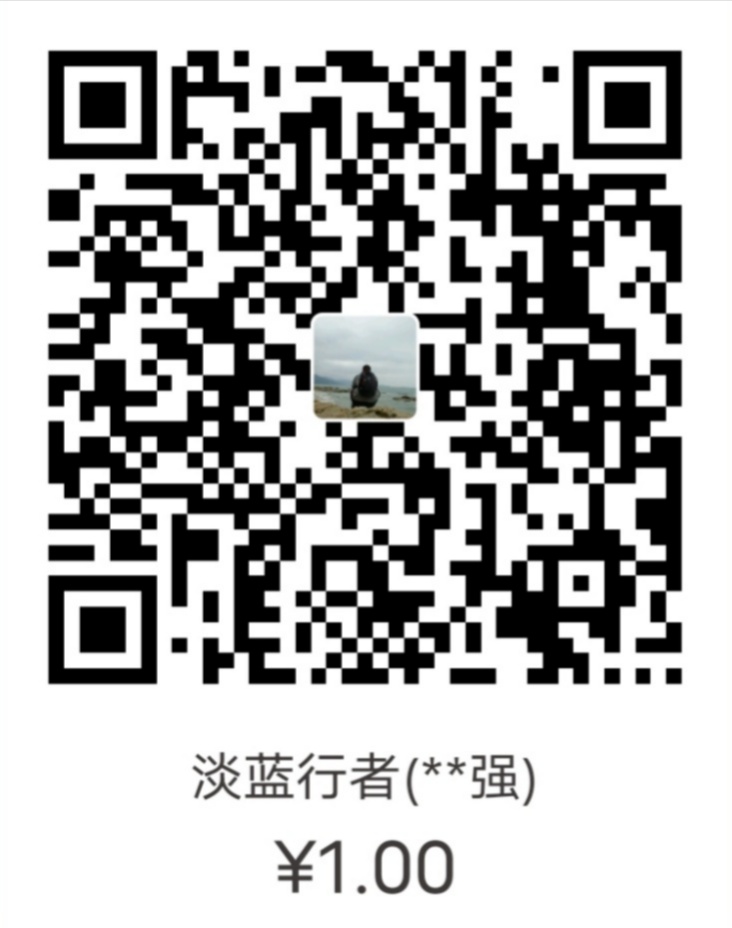