C#托管代码与C++非托管代码互相调使用一(C#调使用C++代码&.net 代码安全)
在最近的项目中,牵涉到项目源代码保密问题,由于代码是C#写的,容易被反编译,因此决定抽取核心算法部分使用C++编写,C++到目前为止好像还不能被很好的反编译,当然如果你是反汇编高手的话,也许还是有可能反编译。这样一来,就涉及C#托管代码与C++非托管代码互相调用,于是调查了一些资料,顺便与大家分享一下
一. C# 中静态调用C++动态链接
1. 建立VC工程CppDemo,建立的时候选择Win32 Console(dll),选择Dll。
2. 在DllDemo.cpp文件中添加这些代码。
extern "C" __declspec(dllexport) int Add(int a,int b)
{
return a+b;
}
3. 编译工程。
4. 建立新的C#工程,选择Console应用程序,建立测试程序InteropDemo
5. 在Program.cs中添加引用:using System.Runtime.InteropServices;
6. 在pulic class Program添加如下代码:
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
namespace InteropDemo
{
class Program
{
[DllImport("CppDemo.dll", EntryPoint = "Add", ExactSpelling = false, CallingConvention = CallingConvention.Cdecl)]
public static extern int Add(int a, int b); //DllImport请参照MSDN
static void Main(string[] args)
{
Console.WriteLine(Add(1, 2));
Console.Read();
}
}
}
好了,现在您可以测试Add程序了,是不是可以在C# 中调用C++动态链接了,当然这是静态调用,需要将CppDemo编译生成的Dll放在DllDemo程序的Bin目录下
二. C# 中动态调用C++动态链接
在第一节中,讲了静态调用C++动态链接,由于Dll路径的限制,使用的不是很方便,C#中我们经常通过配置动态的调用托管Dll,例如常用的一些设计模式:Abstract Factory, Provider, Strategy模式等等,那么是不是也可以这样动态调用C++动态链接呢?只要您还记得在C++中,通过LoadLibrary, GetProcess, FreeLibrary这几个函数是可以动态调用动态链接的(它们包含在kernel32.dll中),那么问题迎刃而解了,下面我们一步一步实验
1. 将kernel32中的几个方法封装成本地调用类NativeMethod
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
namespace InteropDemo
{
public static class NativeMethod
{
[DllImport("kernel32.dll", EntryPoint = "LoadLibrary")]
public static extern int LoadLibrary(
[MarshalAs(UnmanagedType.LPStr)] string lpLibFileName);
[DllImport("kernel32.dll", EntryPoint = "GetProcAddress")]
public static extern IntPtr GetProcAddress(int hModule,
[MarshalAs(UnmanagedType.LPStr)] string lpProcName);
[DllImport("kernel32.dll", EntryPoint = "FreeLibrary")]
public static extern bool FreeLibrary(int hModule);
}
}
2. 使用NativeMethod类动态读取C++Dll,获得函数指针,并且将指针封装成C#中的委托。原因很简单,C#中已经不能使用指针了,如下
int hModule = NativeMethod.LoadLibrary(@"c:"CppDemo.dll");
IntPtr intPtr = NativeMethod.GetProcAddress(hModule, "Add");
详细请参见代码
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
namespace InteropDemo
{
class Program
{
//[DllImport("CppDemo.dll", EntryPoint = "Add", ExactSpelling = false, CallingConvention = CallingConvention.Cdecl)]
//public static extern int Add(int a, int b); //DllImport请参照MSDN
static void Main(string[] args)
{
//1. 动态加载C++ Dll
int hModule = NativeMethod.LoadLibrary(@"c:CppDemo.dll");
if (hModule == 0) return;
//2. 读取函数指针
IntPtr intPtr = NativeMethod.GetProcAddress(hModule, "Add");
//3. 将函数指针封装成委托
Add addFunction = (Add)Marshal.GetDelegateForFunctionPointer(intPtr, typeof(Add));
//4. 测试
Console.WriteLine(addFunction(1, 2));
Console.Read();
}
/// <summary>
/// 函数指针
/// </summary>
/// <param name="a"></param>
/// <param name="b"></param>
/// <returns></returns>
delegate int Add(int a, int b);
}
}
通过如上两个例子,我们可以在C#中动态或者静态的调用C++写的代码了
C#托管代码与C++非托管代码互相调使用二(C++调使用C#代码)
上篇文章提到,目前项目想做到核心部分代码不被反编译,而考虑到团队成员都是比较熟悉C#,因此核心算法部分采用C++,而其他地方则采用C#(例如数据访问层,界面层都使用C#语言)。在上一篇文章中完成了C#托管代码调用C++非托管代码,现在接着完成第二部分,即C++非托管代码调用C#托管代码,分为两部分,首先C#建立COM+组件,其次是C++调用COM+组件。
C#建立COM+组件
1. 在VS中,新建类库ComInterop
2. 在类库新增接口:ComInteropInterface, 及相应的实现ComInterop, ComInterop同时必须继承自ServicedComponent。ComInteropInterface中有两个简单接口:
int Add(int a, int b);
int Minus(int a, int b);
具体代码如下:
using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
using System.Runtime.InteropServices;
using System.EnterpriseServices;
namespace ComInteropDemo
{
//接口声明
[Guid("7103C10A-2072-49fc-AD61-475BEE1C5FBB")]
public interface ComInteropInterface
{
[DispId(1)]
int Add(int a, int b);
[DispId(2)]
int Minus(int a, int b);
}
//对于实现类的声明
[Guid("87796E96-EC28-4570-90C3-A395F4F4A7D6")]
[ClassInterface(ClassInterfaceType.None)]
public class ComInterop : ServicedComponent, ComInteropInterface
{
public ComInterop() { }
public int Add(int a, int b)
{
return a + b;
}
public int Minus(int a, int b)
{
return a - b;
}
}
}
3 . 使用REGASM命令导出虚拟表,当重新编译生产Dll时需要使用REGASM /u命令将前一次Dll注销
REGASM ComInteropDemo.dll /tlb ComInteropDemo.tlb
REGASM /u ComInteropDemo.dll
首先对COM+组件的写法需要注意以下几点:
2. 方法和属性必须在接口中声明,事件也必须在事件接口中声明.
否则将在VC中无法调用,在接口中声明主要是为了在COM 中的vtab中.
3. 必须对接口中的方法,属性,事件前声明[DispId(1)]
5. 而且项目一定需要是COM Interop,并且具有强命名
6. 组件ComVisible属性必须为true,这里强调的原因是VS中默认值为false
C++调用C# COM+组件
步骤:
1. 建立C++ 项目CppLoader,项目类型选择Win32,控制台应用程序
2. 在头文件中导入类型库tlb
#import "..\Debug\ComInteropDemo.tlb"
3. 初始化COM以及产生智能指针(一般是在需要调用COM组件中提供的方法时就需要产生指向该接口的智能指针)
4. 调用COM中的方法Add
5. 释放环境 ,具体代码如下
#include "stdafx.h"
#include <iostream>
using namespace std;
#import "..\Debug\ComInteropDemo.tlb"
//路径一定要正确
int _tmain(int argc, _TCHAR* argv[])
{
HRESULT hr;
//ComInteropDemo::ComInterop *p;
//初始化COM
CoInitialize ( NULL );
//创建智能指针ComInteropDemo::ComInteropInterface
ComInteropDemo::ComInteropInterfacePtr ptr;
//创建实例
hr = ptr.CreateInstance(__uuidof (ComInteropDemo::ComInterop));
if(hr == S_OK)
{
cout << ptr->Add (1.0, 2.0);
}
CoUninitialize ();
return 0;
}
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
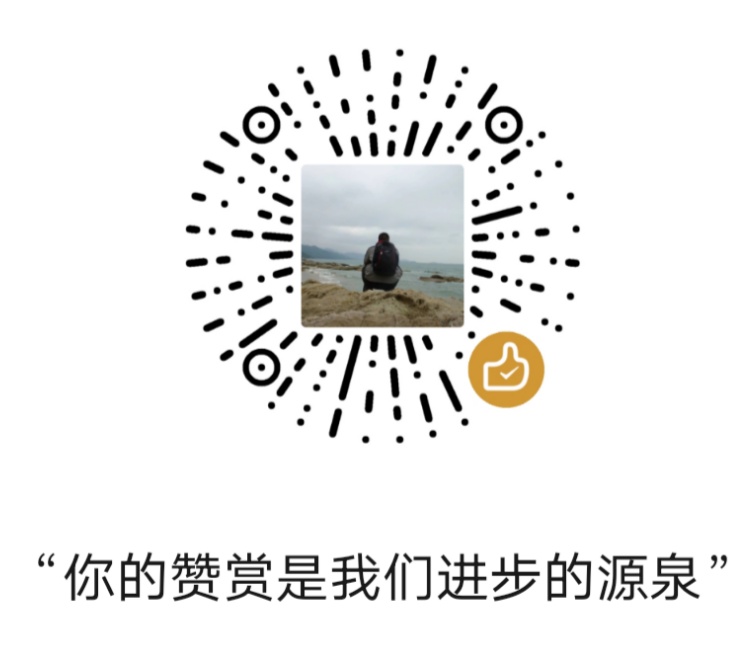
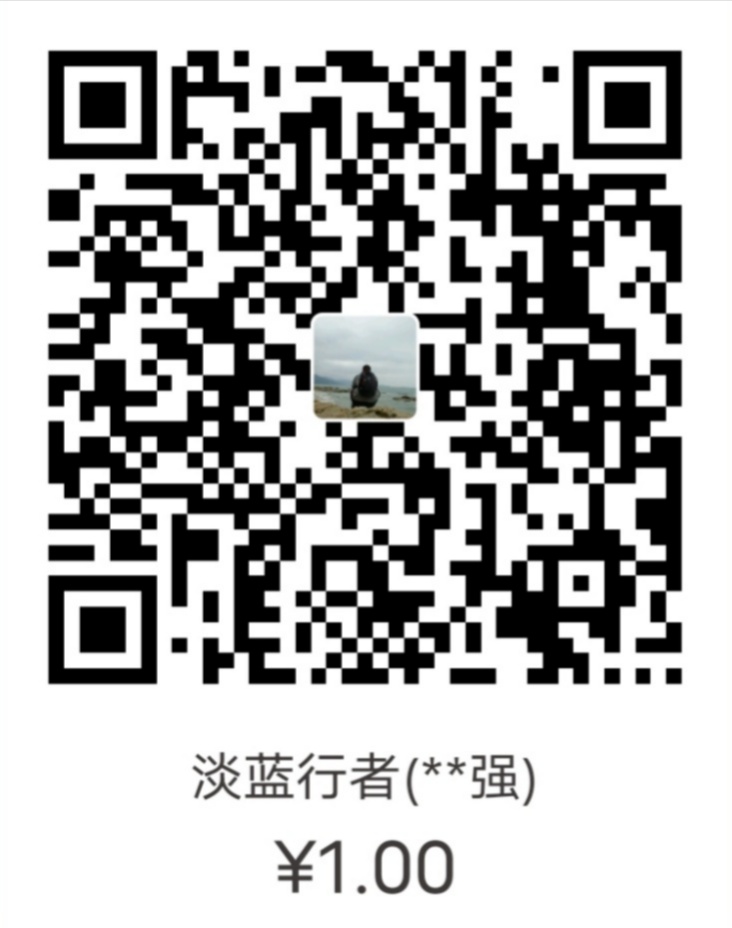