目的:实现发布WebService服务并使用该服务
WebService服务端
构架图
(原图无法显示)
1、创建出项目时 添加 Xfire Web Service
项目属性 -> MyEclipse ->Add Xfire Web Service
(原图无法显示)
这步可以默认 -> 下一步
这时需要 选中 XFire 1.2 HTTP Client Libraries
(原图无法显示)
2、定义接口与实现类
package com.accp.Inter; /** * 定义接口 * @author jaylian * 2009年3月27日13:26:51 */ public interface Ihello { public String hello(); } package com.accp.Impl; /** * 实现类 * @author jaylian * 2009年3月27日13:29:32 */ import com.accp.Inter.Ihello; public class ImplHello implements Ihello { public String hello() { return "Hello Accp !!"; } }
3、services.xml 中的配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://xfire.codehaus.org/config/1.0">
<service>
<name>ACCP</name>
<serviceClass>com.accp.Inter.Ihello</serviceClass>
<implementationClass>com.accp.Impl.ImplHello</implementationClass>
</service>
</beans>
(编辑注:
上述为spring1.x配置
spring2.x为:
<?xml version="1.0" encoding="UTF-8"?> <beans> <service xmlns="http://xfire.codehaus.org/config/1.0"> <name>ACCP</name> <serviceClass>com.accp.Inter.Ihello</serviceClass> <implementationClass>com.accp.Impl.ImplHello</implementationClass> </service> </beans> )
4、启动Tomcat
运行页面: http://localhost:8080/WebService/services/
Available Services:
ACCP [wsdl]
Generated by XFire ( http://xfire.codehaus.org )
5、点击[wsdl] 进入
地址:http://localhost:8080/WebService/services/ACCP?wsdl
<?xml version="1.0" encoding="UTF-8" ?> - <wsdl:definitions targetNamespace="http://Inter.accp.com" xmlns:tns="http://Inter.accp.com" xmlns:wsdlsoap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:soap12="http://www.w3.org/2003/05/soap-envelope" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soapenc11="http://schemas.xmlsoap.org/soap/encoding/" xmlns:soapenc12="http://www.w3.org/2003/05/soap-encoding" xmlns:soap11="http://schemas.xmlsoap.org/soap/envelope/" xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/"> - <wsdl:types> - <xsd:schema xmlns:xsd="http://www.w3.org/2001/XMLSchema" attributeFormDefault="qualified" elementFormDefault="qualified" targetNamespace="http://Inter.accp.com"> - <xsd:element name="hello"> <xsd:complexType /> </xsd:element> - <xsd:element name="helloResponse"> - <xsd:complexType> - <xsd:sequence> <xsd:element maxOccurs="1" minOccurs="1" name="out" nillable="true" type="xsd:string" /> </xsd:sequence> </xsd:complexType> </xsd:element> </xsd:schema> </wsdl:types> - <wsdl:message name="helloRequest"> <wsdl:part name="parameters" element="tns:hello" /> </wsdl:message> - <wsdl:message name="helloResponse"> <wsdl:part name="parameters" element="tns:helloResponse" /> </wsdl:message> - <wsdl:portType name="ACCPPortType"> - <wsdl:operation name="hello"> <wsdl:input name="helloRequest" message="tns:helloRequest" /> <wsdl:output name="helloResponse" message="tns:helloResponse" /> </wsdl:operation> </wsdl:portType> - <wsdl:binding name="ACCPHttpBinding" type="tns:ACCPPortType"> <wsdlsoap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http" /> - <wsdl:operation name="hello"> <wsdlsoap:operation soapAction="" /> - <wsdl:input name="helloRequest"> <wsdlsoap:body use="literal" /> </wsdl:input> - <wsdl:output name="helloResponse"> <wsdlsoap:body use="literal" /> </wsdl:output> </wsdl:operation> </wsdl:binding> - <wsdl:service name="ACCP"> - <wsdl:port name="ACCPHttpPort" binding="tns:ACCPHttpBinding"> <wsdlsoap:address location="http://localhost:8080/WebService/services/ACCP" /> </wsdl:port> </wsdl:service> </wsdl:definitions>
这表示你的WebService发布成了。
6、你还可以再MyEclipse中测试:
(原图无法显示)
最后又输出: out(string): Hello Accp !!
创建WebService客户端
1、New Web Services Project
project Name : WebClient
注意:Framwork:选中中间的 XFire
-> 下一步 默认即可
-> 下一步 选中第二项 XFire 1.2 HTTP Client Libraries
-> 完成
2、创建客户端接口及类
package com.accp.Inter; /** * 客户端接口 * @author jaylian * 2009年3月27日14:03:06 */ public interface Ihello { public String hello(); } package com.accp.Impl; import java.net.MalformedURLException; import org.codehaus.xfire.XFire; import org.codehaus.xfire.XFireFactory; import org.codehaus.xfire.client.XFireProxyFactory; import org.codehaus.xfire.service.Service; import org.codehaus.xfire.service.binding.ObjectServiceFactory; import com.accp.Inter.Ihello; public class HelloWord { public String getHello() { Service serviceModel = new ObjectServiceFactory() .create(Ihello.class); XFire xfire = XFireFactory.newInstance().getXFire(); XFireProxyFactory factory = new XFireProxyFactory(xfire); String serviceUrl = "http://localhost:8080/WebService/services/ACCP"; Ihello client = null; try { client = (Ihello) factory.create(serviceModel, serviceUrl); } catch (MalformedURLException e) { } String result = ""; try { result = client.hello(); } catch (Exception e) { e.printStackTrace(); } System.out.println(result); return result; } public static void main(String[] args) { new HelloWord().getHello(); } }
这里使用了一个Java类,测试获取的webservice服务信息
控制台输出:
Hello Accp !!
(文/D调de恋雨)
本文地址:http://blog.csdn.net/jaylianyu/archive/2009/03/27/4029678.aspx
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
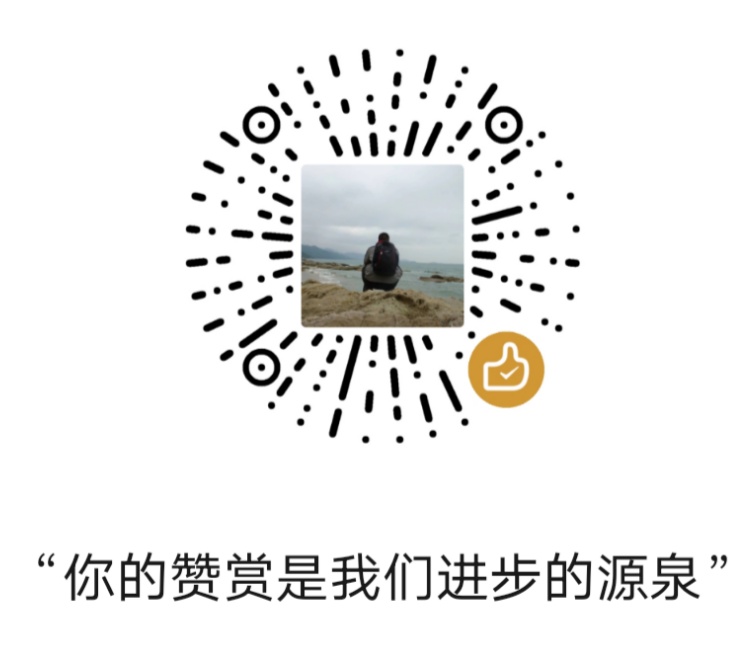
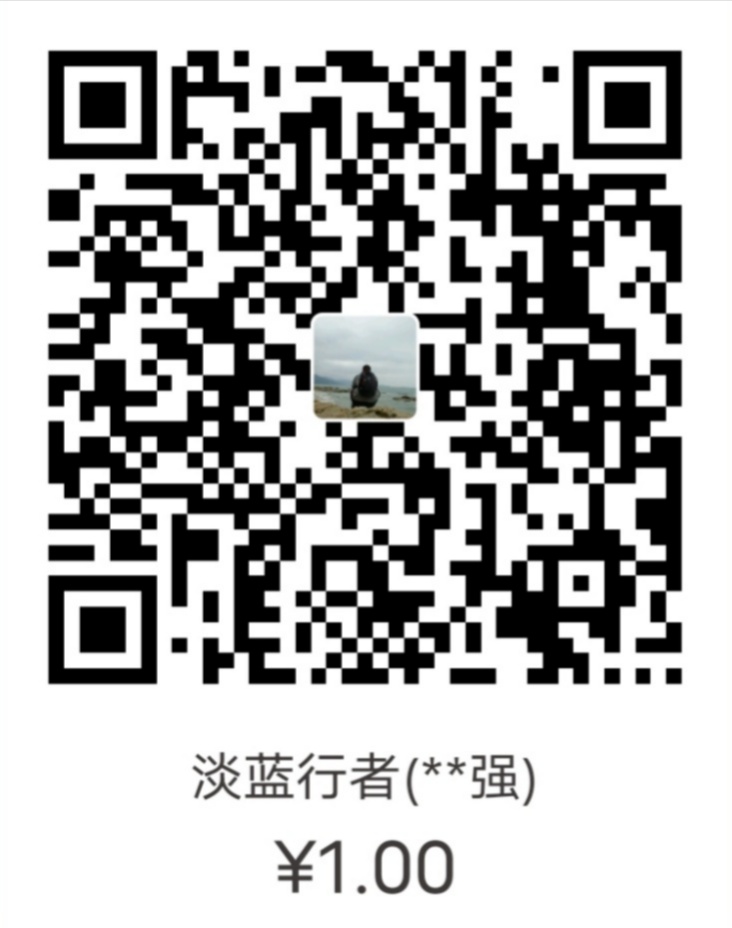