TCP服务端源码示例:
package TCP;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPServer{
public static void main(String[] args) {
ServerSocket ss=null;
Socket s=null;
BufferedReader br=null;
PrintWriter pw=null;
try {
ss=new ServerSocket(7888);
System.out.println("Server is running on port 7888...");
int i=0;
while (true) {
s = ss.accept();
br=new BufferedReader(new InputStreamReader(s.getInputStream()));
i++;
String str=br.readLine();
System.out.println("You are No."+i+" guest, and your IP is:"+s.getInetAddress()+" "+str);
str=str.toUpperCase();
pw = new PrintWriter(s.getOutputStream());
pw.println(str);
pw.flush();
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(pw!=null) pw.close();
if(br!=null)try{br.close();}catch(IOException e){}
if(s!=null)try{s.close();}catch(IOException e){}
if(ss!=null)try{ss.close();}catch(IOException e){}
}
}
}
TCP客户端源码示例:
package TCP;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
public class TCPClient {
public static void main(String[] args) {
Socket s=null;
BufferedReader br=null;
BufferedReader brin=null;
PrintWriter pw=null;
try {
s=new Socket("127.0.0.1",7888);
System.out.println("Please enter something:");
brin=new BufferedReader(new InputStreamReader(System.in));
String strin = brin.readLine();
pw = new PrintWriter(s.getOutputStream());
pw.println(strin);
pw.flush();
br = new BufferedReader(new InputStreamReader(s.getInputStream()));
String str = br.readLine();
System.out.println(str);
} catch (IOException e) {
e.printStackTrace();
}finally {
if(pw!=null) pw.close();
if(brin!=null)try{brin.close();}catch(IOException e){}
if(br!=null)try{br.close();}catch(IOException e){}
if(s!=null)try{s.close();}catch(IOException e){}
}
}
}
另:指定字符编码示例:
String strTest = "TCP测试";
byte[] bTest = strTest.getBytes("UTF-8");
s.getOutputStream().write(bTest);
s.getOutputStream().flush();
byte[] bRst = new byte[1024];
s.getInputStream().read(bRst);
String str = new String(bRst,"UTF-8");
System.out.println(str);
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
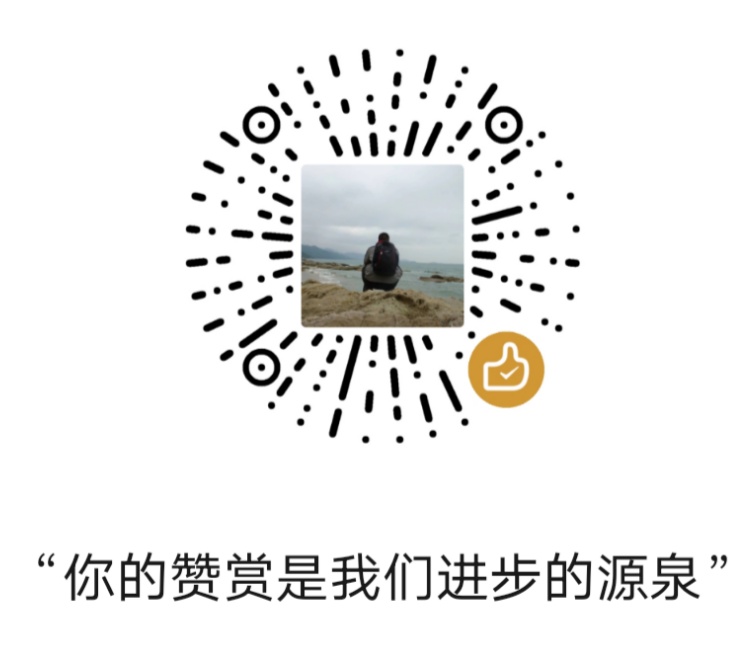
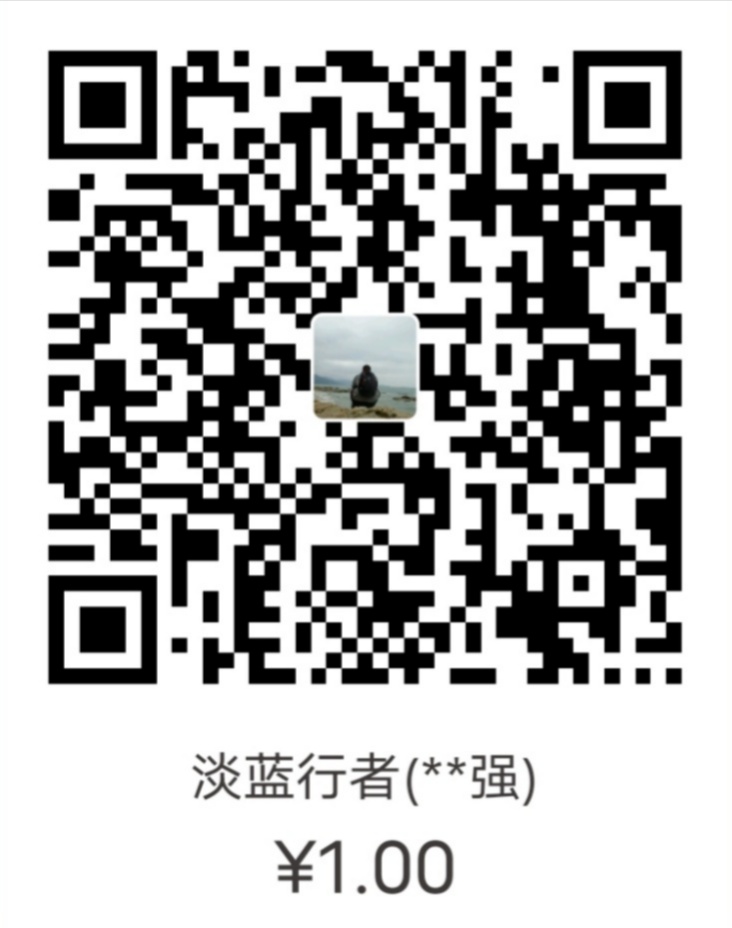