链表实现类(仅供参考):
package link;
public class Link {
private Node head;
public Link(){
head=new Node(null);
}
private static class Node{
public Object data;
public Node next;
public Node(Object obj){
this.data=obj;
//this.next=null;
}
}
/**
* 把 obj 列表加在该链表的末尾
* @param obj
*/
public void append(Object obj){
Node temp=head;
System.out.println("1"+temp.next);
while(temp.next!=null){
// System.out.println("2"+temp.next);
temp=temp.next;
System.out.println("3"+temp.next);
}
Node n=new Node(obj);
temp.next=n;
// System.out.println("4"+n.data);
System.out.println("5"+temp.next.data);
}
/**删除链表中指定的对象
* @return 如果删除成功返回true,如果没有该对象,返回false
*/
public boolean remove(Object obj){
Node temp=head;
while(!temp.next.data.equals(obj)){
System.out.println("1"+temp.next);
temp=temp.next;
if(temp.next==null){
return false;
}
}
temp.next=temp.next.next;
return true;
}
/**
* 在链表的指定位置上插入新的数据
* @param obj 要插入的对象
* @param index 指定的位置
*/
public void insert(Object obj,int index){
Node temp=head;
for(int i=0;i<index;i++){
temp=temp.next;
}
System.out.println("insert"+temp.data);
Node n=new Node(obj);
n.next=temp.next;
temp.next=n;
}
/**
* 删除指定位置上的对象
* @param index 指定的位置
*/
public void remove(int index){
Node temp=head;
for(int i=0;i<index;i++){
temp=temp.next;
}
System.out.println("remove"+temp.data);
temp.next=temp.next.next;
System.out.println("remove"+temp.next.data);
}
}
链表测试类:
package link;
public class LinkTest {
public static void main(String[] args) {
Link lk=new Link();
lk.append("sss");
lk.append("aaa");
lk.append("wqw");
lk.append("yyu");
lk.append("ppp");
lk.append("76y");
lk.remove("wqw");
lk.remove("76y");
lk.insert("insert", 1);
lk.remove(3);
}
}
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
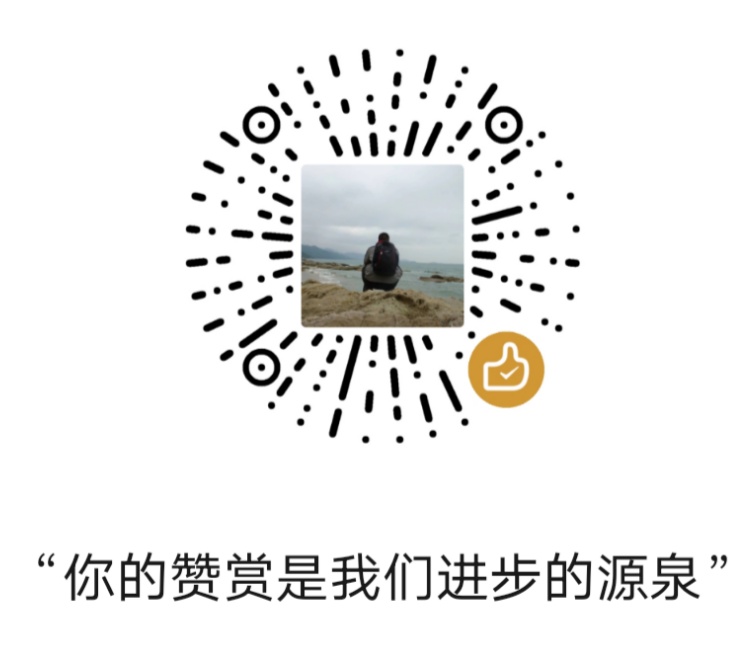
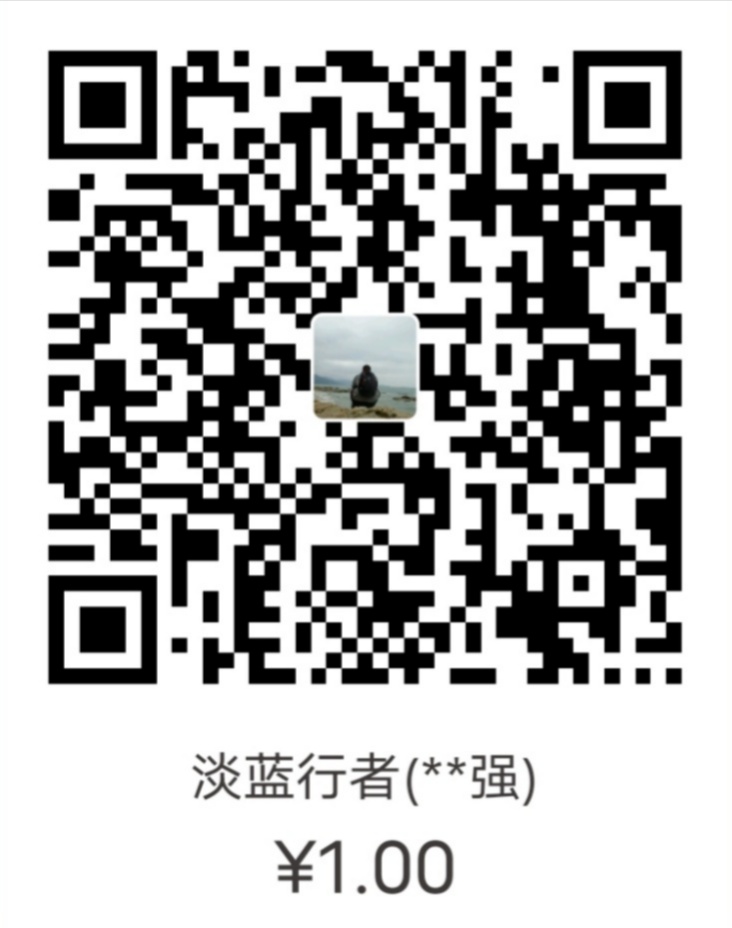