31 Given the following code fragment:
1) String str = null;
2) if ((str != null) && (str.length() > 10)) {
3) System.out.println("more than 10");
4) }
5) else if ((str != null) & (str.length() < 5)) {
6) System.out.println("less than 5");
7) }
8) else { System.out.println("end"); }
Which line will cause error?
A. line 1
B. line 2
C. line 5
D. line 8
(c)
题目:给出下面的代码片断:
…
哪些行将导致错误。
此题需要将代码仔细看清楚,查询没有逻辑错误,if …else的使用没有问题,也没有拼写错误,错误在于第5行的“与”操作符的使用,逻辑操作符(logical operator)的“与”应该是&&,而在执行“与”操作的时候,如果第一个条件为false,那么第二个条件判断运算是不做的,但是这里是位逻辑操作符(bitwise logical operator)的“与”,在进行这个运算时,无论第一个条件的结果是什么都会执行第二个的运算,因此,假设str=null,那么第5句的str.length()就会导致NullPointerException,因此本题的错误在于此。
32、Which statements about Java code security are true?
A. The bytecode verifier loads all classes needed for the execution of a program.
B. Executing code is performed by the runtime interpreter.
C. At runtime the bytecodes are loaded, checked and run in an interpreter.
D. The class loader adds security by separating the namespaces for the classes of the local file system from those imported from network sources.
(bcd)
题目:下面有关java代码安全性的叙述哪些是对的。
A. 字节码校验器加载查询执行需要的所有类。
B. 运行时解释器执行代码。
C. 在运行时,字节码被加载,验证然后在解释器里面运行。
D. 类加载器通过分离本机文件系统的类和从网络导入的类增加安全性。
SL275中描述的Java程序运行的过程是这样的:类加载器(class loader)加载程序运行所需要的所有类,它通过区分本机文件系统的类和网络系统导入的类增加安全性,这可以限制任何的特洛伊木马程序,因为本机类总是先被加载,一旦所有的类被加载完,执行文件的内存划分就固定了,在这个时候特定的内存地址被分配给对应的符号引用,查找表(lookuo table)也被建立,由于内存划分发生在运行时,解释器在受限制的代码区增加保护防止未授权的访问;然后字节码校验器(byte code verifier)进行校验,主要执行下面的检查:类符合JVM规范的类文件格式,没有违反访问限制,代码没有造成堆栈的上溢或者下溢,所有操作代码的参数类型都是正确的,没有非法的数据类型转换(例如将整型数转换成对象类型)发生;校验通过的字节码被解释器(interpreter)执行,解释器在必要时通过运行时系统执行对底层硬件的合适调用。后三个答案是SL275中的原话。
33、Given the following code:
public class Person{
static int arr[] = new int[10];
public static void main(String a[]) {
System.out.println(arr[1];)
}
}
Which statement is correct?
A. When compilation some error will occur.
B. It is correct when compilation but will cause error when running.
C. The output is zero.
D. The output is null.
(c)
题目:给出下面的代码:
…
那个叙述是对的。
A. 编译时将发生错误。
B. 编译时正确但是运行时出错。
C. 输出为0。
D. 输出为null
int型数组是类对象,它在类被加载时完成初始化,在前面题目中已经有叙述,由于是原始数据类型int,其初始值为0。
34、Given the following code:
public class Person{
int arr[] = new int[10];
public static void main(String a[]) {
System.out.println(arr[1]);
}
}
Which statement is correct?
A. When compilation some error will occur.
B. It is correct when compilation but will cause error when running.
C. The output is zero.
D. The output is null.
(a)
给出下面的代码:
…
哪些叙述是对的。
A. 编译时出错。
B. 编译时正确而运行时出错。
C. 输出0。
D. 输出null。
实例变量在类的一个实例构造时完成初始化,而且在类的静态方法中不能直接访问类的非静态成员而只能访问类成员(像上题中一样),类的普通方法可以访问类的所有成员和方法,而静态方法只能访问类的静态成员和方法,因为静态方法属于类,而普通方法及成员变量属于类的实例,类方法(静态方法)不能使用属于某个不确定的类的实例的方法和变量,在静态方法里面没有隐含的this,而普通方法有。
35、public class Parent {
public int addValue( int a, int b) {
int s;
s = a+b;
return s;
}
}
class Child extends Parent {
}
Which methods can be added into class Child?
A. int addValue( int a, int b ){// do something...}
B. public void addValue (){// do something...}
C. public int addValue( int a ){// do something...}
D. public int addValue( int a, int b )throws MyException {//do something...}
(bc)
题目:哪些方法可以加入类Child中。
此题涉及方法重载(overload),方法重写(override)以及类派生时方法重写的规则。方法重载的规则是:一、调用声明的参数列表必须足够不同以便可以无歧义的决定调用合适的重载方法;二、返回值可以不同,但是不能是重载时唯一的不同点(这点和c++中不同,c++中返回类型必须一致)。方法重写发生在类继承时,子类可以重写一个父类中已有的方法,必须在返回类型和参数列表一样时才能说是重写,否则就是重载,java中方法重写的一个重要而且容易被忽略的规则是重写的方法的访问权限不能比被重写的方法的访问权限低!重写的另一个规则是重写的方法不能比被重写的方法抛弃(throws)更多种类的异常,其抛弃的异常只能少,或者是其子类,不能以抛弃异常的个数来判断种类,而应该是异常类层次结果上的种类。此题中答案a的错误就是重写的访问权限比被重写的方法的低,而b,c都属于重载,d的错误在于比被重写的方法抛弃了更多种类的异常。
[NextPage]
36、A member variable defined in a class can be accessed only by the classes in the same package. Which modifier should be used to obtain the access control?
A. private
B. no modifier
C. public
D. protected
(b)
题目:一个类中定义的成员变量只能被同一包中的类访问。下面的哪些修饰符可以获得需要的访问控制。
参看前面的题目中的叙述。
37、A public member vairable called MAX_LENGTH which is int type, the value of the variable remains constant value 100. Use a short statement to define the variable.
A. public int MAX_LENGTH=100;
B. final int MAX_LENGTH=100;
C. final public int MAX_LENGTH=100;
D. public final int MAX_LENGTH=100.
(d)
题目:共有成员变量MAX_LENGTH是一个int型值,变量的值保持常数值100。使用一个短声明定义这个变量。
Java中共有变量使用public定义,常量变量使用final,另外注意的是修饰符的顺序,一个最完整的修饰是public static final int varial_a=100;这个顺序不能错,这和c++中也是不同的。而答案c恰恰错在修饰符的顺序上。
38、Which expressions are correct to declare an array of 10 String objects?
A. char str[];
B. char str[][];
C. String str[];
D. String str[10];
(c)
题目:哪些表达式是声明一个含有10个String对象的数组。
严格来说这个题目没有给出一个正确的答案,唯一比较正确的是c,而完全满足题目要求的应该是:String str[]=new String[10];
注意答案d的形式是不对的,这和c++也是不同的。
39、Which fragments are correct in Java source file?
A. package testpackage;
public class Test{//do something...}
B. import java.io.*;
package testpackage;
public class Test{// do something...}
C. import java.io.*;
class Person{// do something...}
public class Test{// do something...}
D. import java.io.*;
import java.awt.*;
public class Test{// do something...}
(acd)
题目:下面的那个java源文件代码片断是对的。
Java中的package语句必须是源文件中除去说明以外的第一条语句,导入包语句可以有几个,但是必须位于package语句之后,其它类定义之前,一个源文件中可以有几个类,但最多只能有一个是public的,如果有,则源文件的文件名必须和该类的类名相同。
40:
String s= "hello";
String t = "hello";
char c[] = {'h','e','l','l','o'} ;
Which return true?
A. s.equals(t);
B. t.equals(c);
C. s==t;
D. t.equals(new String("hello"));
E. t==c.
(acd)
题目:哪些返回true。
这个在前面第10题的equals()方法和==操作符的讨论中论述过。==操作符比较的是操作符两端的操作数是否是同一个对象,而String的equals()方法比较的是两个String对象的内容是否一样,其参数是一个String对象时才有可能返回true,其它对象都返回假。需要指出的是由于s和t并非使用new创建的,他们指向内存池中的同一个字符串常量,因此其地址实际上是相同的(这个可以从反编译一个简单的测试程序的结果得到,限于篇幅不列出测试代码和反编译的分析),因此答案c也是正确的。
[NextPage]
41、Which of the following statements are legal?
A. long l = 4990;
B. int i = 4L;
C. float f = 1.1;
D. double d = 34.4;
E. double t = 0.9F.
(ade)
题目:下面的哪些声明是合法的。
此题的考点是数字的表示法和基本数据类型的类型自动转换,没有小数点的数字被认为是int型数,带有小数点的数被认为是double型的数,其它的使用在数字后面加一个字母表示数据类型,加l或者L是long型,加d或者D是double,加f或者F是float,可以将低精度的数字赋值给高精度的变量,反之则需要进行强制类型转换,例如将int,short,byte赋值给long型时不需要显式的类型转换,反之,将long型数赋值给byte,short,int型时需要强制转换(int a=(int)123L;)。
42、
public class Parent {
int change() {…}
}
class Child extends Parent {
}
Which methods can be added into class Child?
A. public int change(){}
B. int chang(int i){}
C. private int change(){}
D. abstract int chang(){}
(ab)
题目:哪些方法可被加入类Child。
这个题目的问题在第35题中有详尽的叙述。需要注意的是答案D的内容,子类可以重写父类的方法并将之声明为抽象方法,但是这引发的问题是类必须声明为抽象类,否则编译不能通过,而且抽象方法不能有方法体,也就是方法声明后面不能带上那两个大括号({}),这些D都不能满足。
43、
class Parent {
String one, two;
public Parent(String a, String b){
one = a;
two = b;
}
public void print(){ System.out.println(one); }
}
public class Child extends Parent {
public Child(String a, String b){
super(a,b);
}
public void print(){
System.out.println(one + " to " + two);
}
public static void main(String arg[]){
Parent p = new Parent("south", "north");
Parent t = new Child("east", "west");
p.print();
t.print();
}
}
Which of the following is correct?
A. Cause error during compilation.
B. south
east
C. south to north
east to west
D. south to north
east
E. south
east to west
(e)
题目:下面的哪些正确。
A. 在编译时出错。
这个题目涉及继承时的多态性问题,在前面的问题中已经有讲述,要注意的是语句t.print();在运行时t实际指向的是一个Child对象,即java在运行时决定变量的实际类型,而在编译时t是一个Parent对象,因此,如果子类Child中有父类中没有的方法,例如printAll(),那么不能使用t.printAll()。参见12题的叙述。
44、A Button is positioned in a Frame. Only height of the Button is affected by the Frame while the width is not. Which layout manager should be used?
A. FlowLayout
B. CardLayout
C. North and South of BorderLayout
D. East and West of BorderLayout
E. GridLayout
(d)
题目:一个按钮放在一个框架中,在框架改变时只影响按钮的高度而宽度不受影响,应该使用哪个布局管理器?
这个还是布局管理器的问题,流布局管理器(FlowLayout)将根据框架的大小随时调整它里面的组件的大小,包括高度和宽度,这个管理器不会约束组件的大小,而是允许他们获得自己的最佳大小,一行满了以后将在下一行放置组件;卡片管理器(CardLayout)一次显式一个加入的组件(根据加入时的关键字);网格管理器(GridLayout)将容器划分为固定的网格,容器大小的改变将影响所有组件的大小,每个组件的大小都会同等地变化;边界管理器(BorderLayout)将容器划分为五个区域,分为东南西北和中间,东西区域的宽度为该区域里面组件的最佳宽度,高度为容器的高度减去南北区域的高度,这是一个可能变化的值,而南北区域的宽度为容器的整个宽度,高度为组件的最佳高度,中间区域的高度为容器的高度减去南北区域的高度,宽度为容器的宽度减去东西区域的宽度。
45、Given the following code:
1) class Parent {
2) private String name;
3) public Parent(){}
4) }
5) public class Child extends Parent {
6) private String department;
7) public Child() {}
8) public String getValue(){ return name; }
9) public static void main(String arg[]) {
10) Parent p = new Parent();
11) }
12) }
Which line will cause error?
A. line 3
B. line 6
C. line 7
D. line 8
E. line 10
(d)
题目:给出下面的代码:
…
哪些行将导致错误。
第8行的getValue()试图访问父类的私有变量,错误,参看前面有关变量类型及其作用域的叙述。
[NextPage]
46、The variable "result" is boolean. Which expressions are legal?
A. result = true;
B. if ( result ) { // do something... }
C. if ( result!= 0 ) { // so something... }
D. result = 1
(ab)
题目:变量"result"是一个boolean型的值,下面的哪些表达式是合法的。
Java的boolean不同于c或者c++中的布尔值,在java中boolean值就是boolean值,不能将其它类型的值当作boolean处理。
47、Class Teacher and Student are subclass of class Person.
Person p;
Teacher t;
Student s;
p, t and s are all non-null.
if(t instanceof Person) { s = (Student)t; }
What is the result of this sentence?
A. It will construct a Student object.
B. The expression is legal.
C. It is illegal at compilation.
D. It is legal at compilation but possible illegal at runtime.
(c)
题目:类Teacher和Student都是类Person的子类
…
p,t和s都是非空值
…
这个语句导致的结果是什么
A. 将构造一个Student对象。
B. 表达式合法。
C. 编译时非法。
D. 编译时合法而在运行时可能非法。
instanceof操作符的作用是判断一个变量是否是右操作数指出的类的一个对象,由于java语言的多态性使得可以用一个子类的实例赋值给一个父类的变量,而在一些情况下需要判断变量到底是一个什么类型的对象,这时就可以使用instanceof了。当左操作数是右操作数指出的类的实例或者是子类的实例时都返回真,如果是将一个子类的实例赋值给一个父类的变量,用instanceof判断该变量是否是子类的一个实例时也将返回真。此题中的if语句的判断没有问题,而且将返回真,但是后面的类型转换是非法的,因为t是一个Teacher对象,它不能被强制转换为一个Student对象,即使这两个类有共同的父类。如果是将t转换为一个Person对象则可以,而且不需要强制转换。这个错误在编译时就可以发现,因此编译不能通过。
48、Given the following class:
public class Sample{
long length;
public Sample(long l){ length = l; }
public static void main(String arg[]){
Sample s1, s2, s3;
s1 = new Sample(21L);
s2 = new Sample(21L);
s3 = s2;
long m = 21L;
}
}
Which expression returns true?
A. s1 == s2;
B. s2 == s3;
C. m == s1;
D. s1.equals(m).
(b)
题目:给出下面的类:
…
哪个表达式返回true。
前面已经叙述过==操作符和String的equals()方法的特点,另外==操作符两边的操作数必须是同一类型的(可以是父子类之间)才能编译通过。
49、Given the following expression about List.
List l = new List(6,true);
Which statements are ture?
A. The visible rows of the list is 6 unless otherwise constrained.
B. The maximum number of characters in a line will be 6.
C. The list allows users to make multiple selections
D. The list can be selected only one item.
(ac)
题目:给出下面有关List的表达式:
…
哪些叙述是对的。
A. 在没有其它的约束的条件下该列表将有6行可见。
B. 一行的最大字符数是6
C. 列表将允许用户多选。
D. 列表只能有一项被选中。
List组件的该构造方法的第一个参数的意思是它的初始显式行数,如果该值为0则显示4行,第二个参数是指定该组件是否可以多选,如果值为true则是可以多选,如果不指定则缺省是不能多选。
50、Given the following code:
class Person {
String name,department;
public void printValue(){
System.out.println("name is "+name);
System.out.println("department is "+department);
}
}
public class Teacher extends Person {
int salary;
public void printValue(){
// doing the same as in the parent method printValue()
// including print the value of name and department.
System.out.println("salary is "+salary);
}
}
Which expression can be added at the "doing the same as..." part of the method printValue()?
A. printValue();
B. this.printValue();
C. person.printValue();
D. super.printValue().
(d)
题目:给出下面的代码:
…
下面的哪些表达式可以加入printValue()方法的"doing the same as..."部分。
子类可以重写父类的方法,在子类的对应方法或其它方法中要调用被重写的方法需要在该方法前面加上”super.”进行调用,如果调用的是没有被重写的方法,则不需要加上super.进行调用,而直接写方法就可以。这里要指出的是java支持方法的诠榈饔茫虼舜鸢竌和b在语法上是没有错误的,但是不符合题目代码中说明处的要求:即做和父类的方法中相同的事情??打印名字和部门,严格来说也可以选a和b。
[NextPage]
51、Which is not a method of the class InputStream?
A. int read(byte[])
B. void flush()
C. void close()
D. int available()
(b)
题目:下面哪个不是InputStream类中的方法
这个题目没有什么好说的,要求熟悉java API中的一些基本类,题目中的InputStream是所有输入流的父类,所有要很熟悉,参看JDK的API文档。方法void flush()是缓冲输出流的基本方法,作用是强制将流缓冲区中的当前内容强制输出。
52、Which class is not subclass of FilterInputStream?
A. DataInputStream
B. BufferedInputStream
C. PushbackInputStream
D. FileInputStream
(d)
题目:
哪个不是FilterInputStream的子类。
此题也是要求熟悉API基础类。Java基础API中的FilterInputStream 的已知子类有:BufferedInputStream, CheckedInputStream, CipherInputStream, DataInputStream, DigestInputStream, InflaterInputStream, LineNumberInputStream, ProgressMonitorInputStream, PushbackInputStream 。
53、Which classes can be used as the argument of the constructor of the class FileInputStream?
A. InputStream
B. File
C. FileOutputStream
D. String
(bd)
题目:哪些类可以作为FileInputStream类的构造方法的参数。
此题同样是要求熟悉基础API,FileInputStream类的构造方法有三个,可接受的参数分别是:File、FileDescriptor、String类的一个对象。
54、Which classes can be used as the argument of the constructor of the class FilterInputStream?
A. FilterOutputStream
B. File
C. InputStream
D. RandomAccessFile
(c)
题目:哪些类可以作为FilterInputStream类的构造方法的参数。
FilterInputStream的构造方法只有一个,其参数是一个InputStream对象。
55、Given the following code fragment:
1) switch(m)
2) { case 0: System.out.println("case 0");
3) case 1: System.out.println("case 1"); break;
4) case 2:
5) default: System.out.println("default");
6) }
Which value of m would cause "default" to be the output?
A. 0
B. 1
C. 2
D. 3
(cd)
题目:给出下面的代码片断:
…
m为哪些值将导致"default"输出。
此题考察switch语句的用法,switch的判断的条件必须是一个int型值,也可以是byte、short、char型的值,case中需要注意的是一个case后面一般要接一个break语句才能结束判断,否则将继续执行其它case而不进行任何判断,如果没有任何值符合case列出的判断,则执行default的语句,default是可选的,可以没有,如果没有default而又没有任何值匹配case中列出的值则switch不执行任何语句。
[NextPage]
56、Given the uncompleted method:
1)
2) { success = connect();
3) if (success==-1) {
4) throw new TimedOutException();
5) }
6)}
TimedOutException is not a RuntimeException. Which can complete the method of declaration when added at line 1?
A. public void method()
B. public void method() throws Exception
C. public void method() throws TimedOutException
D. public void method() throw TimedOutException
E. public throw TimedOutException void method()
(bc)
题目:给出下面的不完整的方法:
…
TimedOutException 不是一个RuntimeException。下面的哪些声明可以被加入第一行完成此方法的声明。
如果程序在运行的过程中抛出异常,而这个异常又不是RuntimeException或者Error,那么程序必须捕获这个异常进行处理或者声明抛弃(throws)该异常,捕获异常可以使用try{}catch(){}语句,而抛弃异常在方法声明是声明,在方法的声明后面加上throws XxxxException,抛弃多个异常时在各异常间使用逗号(,)分隔,题目中的程序在运行时抛出的不是一个RuntimeException,所有必须捕获或者抛弃,而程序又没有捕获,所有应该在方法声明中声明抛弃。由于Exception是所有异常的父类,所有当然也可以代表RuntimeException了。
57、Which of the following answer is correct to express the value 10 in hexadecimal number?
A. 0xA
B. 0x16
C. 0A
D. 016
(a)
题目:下面的哪些答案可以正确表示一个十六进制数字10。
十六进制数以0x开头,以0开头的是八进制数。十六进制表示中的a,b,c,d,e,f依次为10,11,12,13,14,15。
58、Which statements about thread are true?
A. Once a thread is created, it can star running immediately.
B. To use the start() method makes a thread runnable, but it does not necessarily start immediately.
C. When a thread stops running because of pre-emptive, it is placed at the front end of the runnable queue.
D. A thread may cease to be ready for a variety of reasons.
(bd)
题目:有关线程的哪些叙述是对的。
A. 一旦一个线程被创建,它就立即开始运行。
B. 使用start()方法可以使一个线程成为可运行的,但是它不一定立即开始运行。
C. 当一个线程因为抢先机制而停止运行,它被放在可运行队列的前面。
D. 一个线程可能因为不同的原因停止(cease)并进入就绪状态。
一个新创建的线程并不是自动的开始运行的,必须调用它的start()方法使之将线程放入可运行态(runnable state),这只是谖蹲鸥孟叱炭晌狫VM的线程调度程序调度而不是意味着它可以立即运行。线程的调度是抢先式的,而不是分时间片式的。具有比当前运行线程高优先级的线程可以使当前线程停止运行而进入就绪状态,不同优先级的线程间是抢先式的,而同级线程间是轮转式的。一个线程停止运行可以是因为不同原因,可能是因为更高优先级线程的抢占,也可能是因为调用sleep()方法,而即使是因为抢先而停止也不一定就进入可运行队列的前面,因为同级线程是轮换式的,它的运行可能就是因为轮换,而它因抢占而停止后只能在轮换队列中排队而不能排在前面。
59、The method resume() is responsible for resuming which thread's execution?
A. The thread which is stopped by calling method stop()
B. The thread which is stopped by calling method sleep()
C. The thread which is stopped by calling method wait()
D. The thread which is stopped by calling method suspend()
(d)
题目:方法resume()负责恢复哪些线程的执行。
A. 通过调用stop()方法而停止的线程。
B. 通过调用sleep()方法而停止运行的线程。
C. 通过调用wait()方法而停止运行的线程。
D. 通过调用suspend()方法而停止运行的线程。
Thread的API文档中的说明是该方法恢复被挂起的(suspended)线程。该方法首先调用该线程的无参的checkAccess() 方法,这可能在当前线程上抛出SecurityException 异常,如果该线程是活着的(alive)但是被挂起(suspend),它被恢复并继续它的执行进程。
60、Given the following code:
1) public class Test {
2) int m, n;
3) public Test() {}
4) public Test(int a) { m=a; }
5) public static void main(String arg[]) {
6) Test t1,t2;
7) int j,k;
8) j=0; k=0;
9) t1=new Test();
10) t2=new Test(j,k);
11) }
12) }
Which line would cause one error during compilation?
A. line 3
B. line 5
C. line 6
D. line 10
(d)
题目:给出下面的代码:
…
在编译时哪行将导致一个错误。
第10行的声明调用一个带两个参数的Test的构造方法,而该类没有这样的构造方法。
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
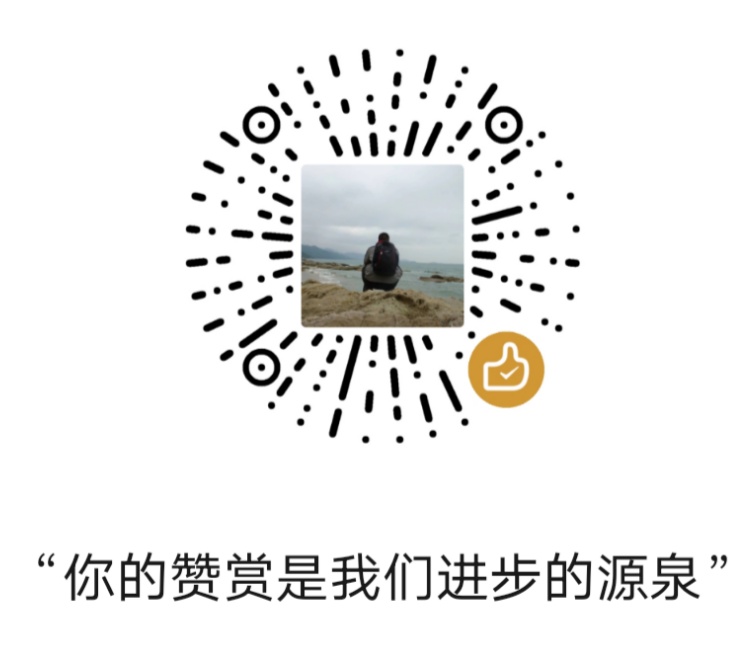
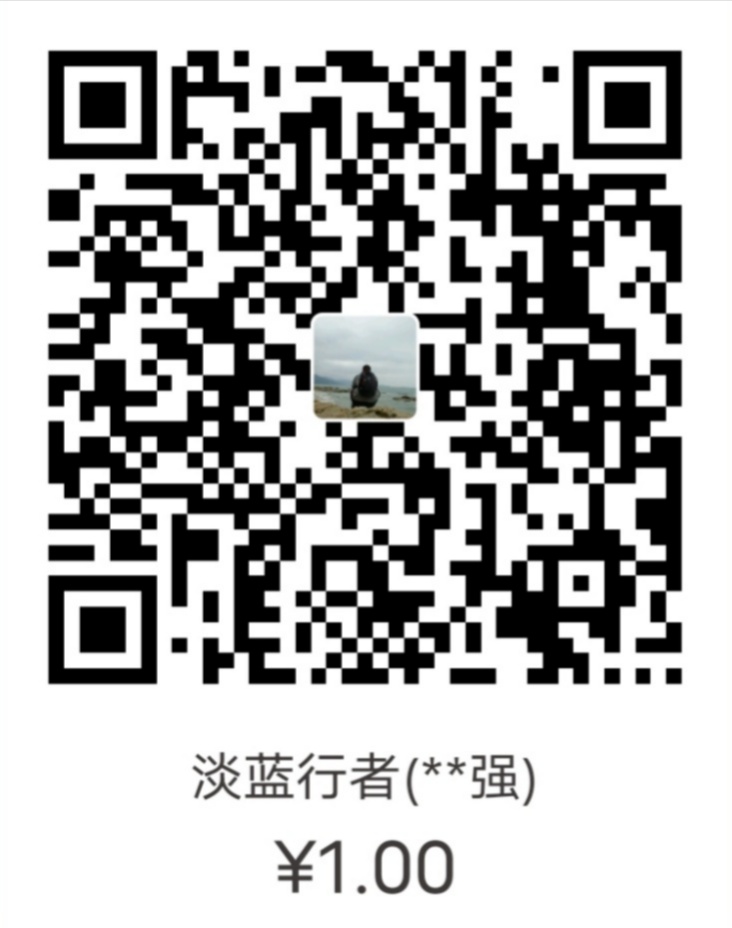