Transact-SQL(又称 T-SQL),是在 Microsoft SQL Server 和 Sybase SQL Server 上的 ANSI SQL 实现,与 Oracle 的 PL/SQL 性质相近(不只是实现 ANSI SQL,也为自身数据库系统的特性提供实现支持),目前在 Microsoft SQL Server和 Sybase Adaptive Server中仍然被使用为核心的查询语言。
Transact-SQL 是具有批量与区块特性的 SQL 指令集合,数据库开发人员可以利用它来撰写数据部份的商业逻辑 (Data-based Business Logic),以强制限制前端应用程序对数据的控制能力。同时,它也是数据库对象的主要开发语言。
语言结构
Transact-SQL 以 ANSI SQL 为主要组成,目前 Microsoft 实现的 Transact-SQL 可支持到 ANSI SQL-92 标准。
ANSI SQL 基础语法支持
DDL
DDL (Data Definition Language) 是对于数据库对象的控制语法,对数据库对象(如数据表,预存程序,函数或自定义类型等)的新增,修改和删除都使用此语法。
CREATE(创建数据库对象)
ALTER(修改数据库对象)
DROP(删除数据库对象)
DML
DML (Data Manipulation Language) 是一般开发人员俗称的 CRUD (Create/Retrieve/Update/Delete) 功能,意指数据的新增/截取/修改/删除四个功能。
SELECT (R)
INSERT (C)
UPDATE (U)
DELETE (D)
DCL
DCL (Data Control Language) 是由数据库所提供的保安功能,对于数据库与数据库对象的访问原则与权限,都由 DCL 定义之。
GRANT(赋与权限)
REVOKE(撤消权限)
批量
Transact-SQL 可以使用分号 ";" 来分区不同的 SQL 指令。例如:
INSERT INTO myTable (myText) VALUES (@myText); SELECT @@IDENTITY
控制流语法
Transact-SQL 可支持下列的控制流程语法 (control-flow):
1 BEGIN ... END,标示 SQL 指令区块,使用 BEGIN ... END 包装的指令会被视为同一个指令区块。
2 IF ... ELSE 的条件式,并可支持嵌套式的 IF 判断式,若 IF 或 ELSE 中的指令包含两个以上,则必须要使用 BEGIN ... END 来标示区块,否则会发生语法检查错误。
3 WHILE 循环,这也是 Transact-SQL 中唯一支持的循环,循环中的指令要用 BEGIN...END 包装。
4 RETURN,可强制终止区块的运行。
5 WAITFOR,可强制让陈述式等待指定时间后才继续运行。
6 GOTO,可导向运行指令到指定的位置。
自定义变量
在 Transact-SQL 中,可以利用 DECLARE 来声明变量,用 SET 来设置变量值,用 SELECT @var = column 的方式,由一个陈述式的回传值中来取得变量值。
DECLARE @v int -- declare a variable
SET @v = 50 -- set variable directly.
SELECT @v = SUM(Qty) FROM SaleItemRecords WHERE SaleID = 53928 -- set variable from a result of statement
错误处理
Transact-SQL 可以在区块中使用下列方式来处理或引发错误:
1 RAISERROR,掷出自定义的错误状况。
2 TRY ... CATCH,使用结构化的方式来处理错误(只有 Microsoft SQL Server 实现的 Transact-SQL 支持)。
3 PRINT,可以印出变量值。
开发与管理工具
Transact-SQL 通常会使用由 SQL Server 或 Adaptive Server 提供的查询工具,像是:
SQL Server Query Analyzer (SQL Server 2000, 7.0, 6.5)
SQL Server Management Studio (SQL Server 2008(也可用 Visual Studio), 2005)
osql.exe,SQL Server 2000, 7.0 的命令行 SQL 工具。
sqlcmd.exe,SQL Server 2005 的命令行 SQL 工具
参考资料
1 Transact-SQL Reference
2 Sybase Transact-SQL User's Guide
Transact-SQL (T-SQL) is Microsoft's and Sybase's proprietary extension to SQL. SQL, often expanded to Structured Query Language, is a standardized computer language that was originally developed by IBM for querying, altering and defining relational databases, using declarative statements. T-SQL expands on the SQL standard to include procedural programming, local variables, various support functions for string processing, date processing, mathematics, etc. and changes to the DELETE and UPDATE statements. These additional features make Transact-SQL Turing complete.
Transact-SQL is central to using Microsoft SQL Server. All applications that communicate with an instance of SQL Server do so by sending Transact-SQL statements to the server, regardless of the user interface of the application.
Flow control
Keywords for flow control in Transact-SQL include BEGIN and END, BREAK, CONTINUE, GOTO, IF and ELSE, RETURN, WAITFOR, and WHILE.
IF and ELSE allow conditional execution. This batch statement will print "It is the weekend" if the current date is a weekend day, or "It is a weekday" if the current date is a weekday.
IF DATEPART(dw, GETDATE()) = 7 OR DATEPART(dw, GETDATE()) = 1
PRINT 'It is the weekend.'
ELSE
PRINT 'It is a weekday.'
BEGIN and END mark a block of statements. If more than one statement is to be controlled by the conditional in the example above, we can use BEGIN and END like this:
IF DATEPART(dw, GETDATE()) = 7 OR DATEPART(dw, GETDATE()) = 1
BEGIN
PRINT 'It is the weekend.'
PRINT 'Get some rest!'
END
ELSE
BEGIN
PRINT 'It is a weekday.'
PRINT 'Get to work!'
END
WAITFOR will wait for a given amount of time, or until a particular time of day. The statement can be used for delays or to block execution until the set time.
RETURN is used to immediately return from a stored procedure or function.
BREAK ends the enclosing WHILE loop, while CONTINUE causes the next iteration of the loop to execute. An example of a WHILE loop is given below.
DECLARE @i NVARCHAR(50)
SET @i = 0
WHILE @i < 5
BEGIN
PRINT 'Hello world.'
SET @i = @i + 1
END
Changes to DELETE and UPDATE statements
In Transact-SQL, both the DELETE and UPDATE statements allow a FROM clause to be added, which allows joins to be included.
This example deletes all users who have been flagged with the 'Idle' flag.
DELETE users
FROM users AS u
JOIN user_flags AS f
ON u.id=f.id
WHERE f.name = 'Idle'
BULK INSERT
BULK INSERT is a Transact-SQL statement that implements a bulk data-loading process, inserting multiple rows into a table, reading data from an external sequential file. Use of BULK INSERT results in better performance than processes that issue individual INSERT statements for each row to be added. Additional details are available on Microsoft's MSDN page.
See also
Adaptive Server Enterprise (Sybase)
PL/SQL (Oracle)
SQL (ANSI)
SQL Server (Microsoft)
External links
Sybase Transact-SQL User's Guide
Transact-SQL Reference for SQL Server 2000 (MSDN)
Transact-SQL Reference for SQL Server 2005 (MSDN)
Transact-SQL Reference for SQL Server 2008 (MSDN)
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
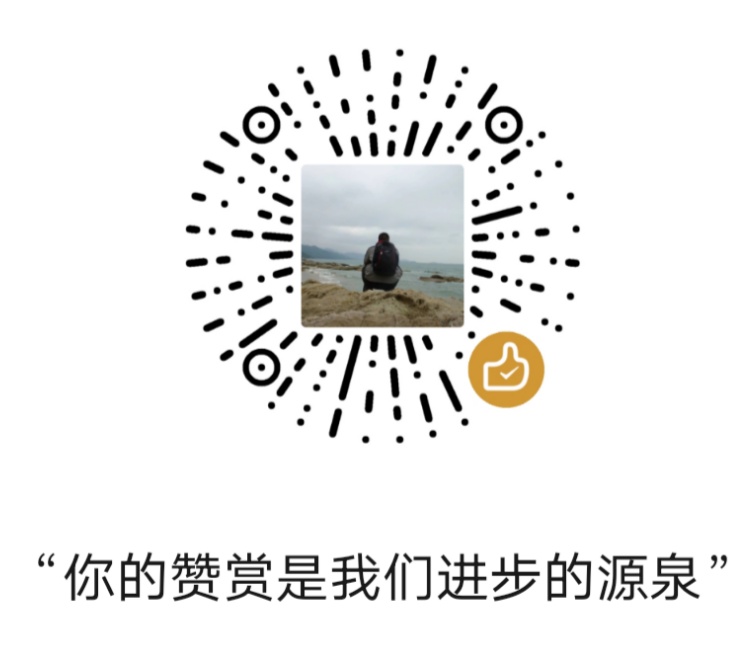
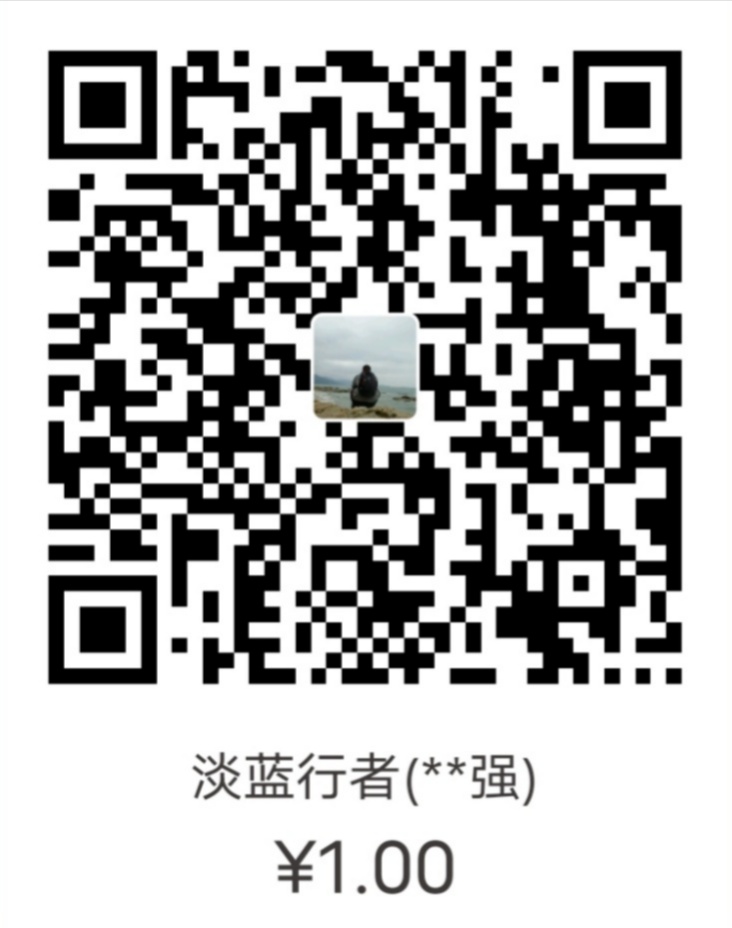