最近一个项目需要调用一个webservice接口,遇到一个问题。项目中的jdom版本为0.9,而webservice client需要用到jdom1.0。如果将jdom版本升级则会造成现有系统异常。
因此需要在不改变现有项目jar的情况下解决这个问题。service端使用的jax-ws2。
wsdl如下:
<?xml version="1.0" encoding="UTF-8"?><!-- Published by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.1.1 in JDK 6. --><!-- Generated by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.1.1 in JDK 6. --><definitions xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:tns="http://www.hua-xia.com.cn/ZySearch" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns="http://schemas.xmlsoap.org/wsdl/" targetNamespace="http://www.hua-xia.com.cn/ZySearch" name="UserLinkWebServiceService">
<types></types>
<message name="getUserLink">
<part name="linkNo" type="xsd:string"></part>
</message>
<message name="getUserLinkResponse">
<part name="returnVal" type="xsd:string"></part>
</message>
<portType name="UserLinkWebService">
<operation name="getUserLink" parameterOrder="linkNo">
<input message="tns:getUserLink"></input>
<output message="tns:getUserLinkResponse"></output>
</operation>
</portType>
<binding name="UserLinkWebServicePortBinding" type="tns:UserLinkWebService">
<soap:binding transport="http://schemas.xmlsoap.org/soap/http" style="rpc"></soap:binding>
<operation name="getUserLink">
<soap:operation soapAction="getUserLink"></soap:operation>
<input>
<soap:body use="literal" namespace="http://www.hua-xia.com.cn/ZySearch"></soap:body>
</input>
<output>
<soap:body use="literal" namespace="http://www.hua-xia.com.cn/ZySearch"></soap:body>
</output>
</operation>
</binding>
<service name="UserLinkWebServiceService">
<port name="UserLinkWebServicePort" binding="tns:UserLinkWebServicePortBinding">
<soap:address location="http://192.168.1.1.154:9010/ZySearch"></soap:address>
</port>
</service>
</definitions>
1.xfire调用
对方给我们的client是使用xfire的client调用,代码如下:
Java代码
package com.zhongying.bussserv.common;
import java.net.URL;
import java.util.Map;
import org.codehaus.xfire.client.Client;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
public class Test {
public static void main(String[] args) {
String url = "http://192.168.1.1:8000/RES_Searcher/service/IUserLinkWebService?wsdl";
Client client;
try {
client = new Client(new URL(url));
Object params[] = {"123456"};
String result = (String)client.invoke("getUserLink", params)[0];
XStream xStream = new XStream(new DomDriver());
Map map = (Map)xStream.fromXML(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
2.axis调用
但是由于jar包的原因,我们不能使用上面的方法,想出的第一个解决方案是使用axis的客户端来调用接口,代码如下:
Java代码
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import javax.xml.namespace.QName;
import org.apache.axis.client.Call;
import org.apache.axis.client.Service;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
public class TestReflection {
public static void main(String[] arg) {
String url = "http://192.168.1.1:9010/ZySearch";
try {
Service service = new Service();
Call call = (Call) service.createCall();
// 设置调用服务地址
call.setTargetEndpointAddress(new java.net.URL(url));
//此处一定要配置wsdl的namespace参数http://www.hua-xia.com.cn/ZySearch
call.setOperationName(new QName("http://www.hua-xia.com.cn/ZySearch", "getUserLink"));
//此处需要配置传入参数类型与参数名称,如果未设置jax-ws则无法接受参数,会认为传入的参数为null
call.addParameter("linkNo",org.apache.axis.encoding.XMLType.XSD_STRING,javax.xml.rpc.ParameterMode.IN);
//如果设置类传入参数类型,此处也需要设置返回参数类型
call.setReturnType(org.apache.axis.encoding.XMLType.XSD_STRING);
call.setUseSOAPAction(true);
call.setSOAPActionURI(url);
String result = (String) call.invoke(new Object[] { "AD0006526305" });
XStream xStream = new XStream(new DomDriver());
Map map = (Map) xStream.fromXML(result);
Iterator it = map.entrySet().iterator();
while (it.hasNext()) {
Map.Entry enty = (Entry) it.next();
System.out.println(enty.getKey() + ":" + enty.getValue());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
3.http模拟调用
由于开始对axis的了解有限,在写axis调用是总是有问题,于是便想了另外一个方法,这个方法有点剑走偏锋。但是适用性却很强,因为调用的是java自带的api不会产生兼容性问题。
大家知道webservice请求实际上也就是一个http请求,将数据通过xml文件进行交换。
既然知道了原理我们就可以模拟一个http请求来调用webservice。
Java代码
try{
String url2 = "http://192.168.1.1:9010/ZySearch?wsdl";
URL getUrl = new URL(url2);
HttpURLConnection connection = (HttpURLConnection) getUrl.openConnection();
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-type","text/xml");
connection.setRequestProperty("Accept","text/xml");
connection.setRequestProperty("User-Agent","JAX-WS RI 2.1.3-hudson-390-");
String send="<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/\" xmlns:xsd="http://www.w3.org/2001/XMLSchema\" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"><soap:Body><ns1:getUserLink xmlns:ns1="http://www.hua-xia.com.cn/ZySearch"><linkNo xmlns="">"+par+"</linkNo></ns1:getUserLink></soap:Body></soap:Envelope>";
connection.getOutputStream().write(send.getBytes());
connection.getOutputStream().flush();
connection.getOutputStream().close();
connection.connect();
// 取得输入流,并使用Reader读取
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream(),"utf-8"));//设置编码,否则中文乱码
System.out.println("=============================");
System.out.println("Contents of get request");
System.out.println("=============================");
String lines="";
String reuslts = "";
while ((lines = reader.readLine()) != null){
//lines = new String(lines.getBhqytes(), "utf-8");
reuslts+=lines.replaceAll("<", "<").replaceAll(">", ">");
}
reader.close();
System.out.println("type:"+connection.getContentType());
// 断开连接
connection.disconnect();
System.out.println(reuslts);
System.out.println("=============================");
System.out.println("Contents of get request ends");
System.out.println("=============================");
XStream xStream = new XStream(new DomDriver());
reuslts = reuslts.substring(reuslts.indexOf("<returnVal>")+11);
reuslts = reuslts.substring(0,reuslts.indexOf("</returnVal>"));
System.out.println(reuslts);
Map result = (Map)xStream.fromXML(reuslts);
Iterator it = result.entrySet().iterator();
while(it.hasNext()){
Map.Entry enty = (Entry) it.next();
System.out.println(enty.getKey()+":"+enty.getValue());
}
}catch(Exception e){
e.printStackTrace();
}
4.jax-ws调用
最后再写上一个jax-ws的client代码
Java代码
import java.net.MalformedURLException;
import java.util.Map;
import javax.xml.namespace.QName;
import javax.xml.soap.MessageFactory;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPBodyElement;
import javax.xml.soap.SOAPElement;
import javax.xml.soap.SOAPMessage;
import javax.xml.ws.BindingProvider;
import javax.xml.ws.Dispatch;
import javax.xml.ws.Service;
import javax.xml.ws.WebServiceException;
import javax.xml.ws.soap.SOAPBinding;
public class Client {
// 名字空间
public static final String targetNamespace = "http://www.hua-xia.com.cn/ZySearch";
//服务名
public static final String serName = "UserLinkWebServiceService";
//端口名
public static final String pName = "UserLinkWebServicePort";
//服务地址
public static final String endpointAddress = "http://192.168.1.1:9010/ZySearch?wsdl";
//方法名
public static final String OPER_NAME = "getUserLink";
//参数名
public static final String INPUT_NMAE = "linkNo";
public static void main(String[] args) throws MalformedURLException, Exception {
// TODO Auto-generated method stub
QName serviceName = new QName(targetNamespace, serName);
QName portName = new QName(targetNamespace, pName);
javax.xml.ws.Service service = Service.create(serviceName);
service.addPort(portName, SOAPBinding.SOAP11HTTP_BINDING, endpointAddress);
Dispatch<SOAPMessage> dispatch = service.createDispatch(portName,
SOAPMessage.class, Service.Mode.MESSAGE);
BindingProvider bp = (BindingProvider) dispatch;
Map<String, Object> rc = bp.getRequestContext();
rc.put(BindingProvider.SOAPACTION_USE_PROPERTY, Boolean.TRUE);
rc.put(BindingProvider.SOAPACTION_URI_PROPERTY, OPER_NAME);
MessageFactory factory = ((SOAPBinding)bp.getBinding()).getMessageFactory();
SOAPMessage request = factory.createMessage();
SOAPBody body = request.getSOAPBody();
QName payloadName = new QName(targetNamespace, OPER_NAME, "ns1");
SOAPBodyElement payload = body.addBodyElement(payloadName);
SOAPElement message = payload.addChildElement(INPUT_NMAE);
message.addTextNode("AD0006526305");
SOAPMessage reply = null;
try
{
reply = dispatch.invoke(request);
}
catch (WebServiceException wse)
{
wse.printStackTrace();
}
SOAPBody soapBody = reply.getSOAPBody();
SOAPBodyElement nextSoapBodyElement = (SOAPBodyElement)soapBody.getChildElements().next ();
SOAPElement soapElement = (SOAPElement)nextSoapBodyElement.getChildElements().next();
System.out.println("获取回应信息为:" + soapElement.getValue());
}
}
(文/按图索骥)
本文来源:http://blog.sina.com.cn/s/blog_69d0d3310100pmec.html
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
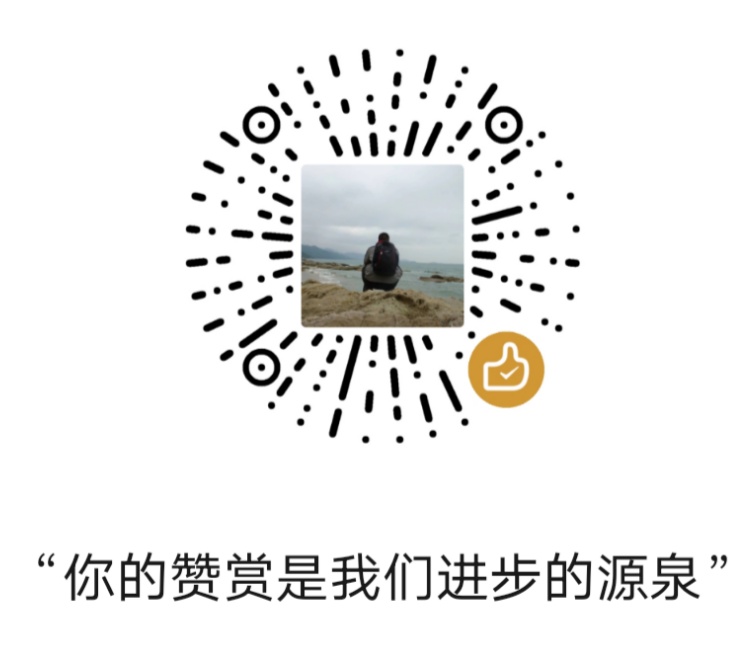
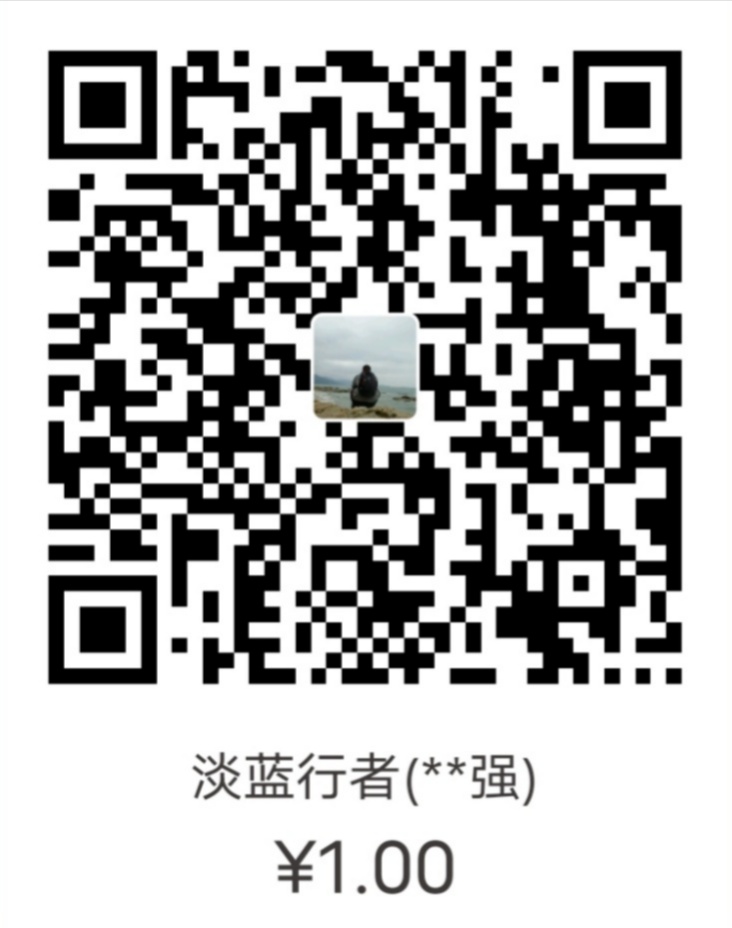