The best recipe for becoming a complete flop in Google Play is to release an app that is battery and memory hungry with a slow interface. Most likely, these qualities will ensure negative reviews from users and result in a bad reputation, even if your app has great design and a unique idea.
Every drawback in product efficiency, battery and memory consumption can really affect your app’s success. That’s why it is critical to develop well-optimized, smooth running apps that never make Android system guard against them. We will not speak about efficient coding, since it goes without saying that the code you write should stand any performance test. But even brilliant code takes time to run. In today’s post, we’ll talk about how to minimize this time and make Android apps that users love.
Efficient threading
Tip 1. How to off-load operations onto threads in background
Since by default all operations in an app run on the main thread (UI thread) in the foreground, the app responsiveness might be affected, which will imminently result in hangs, freezes and even system errors.
To reinforce responsiveness, you should shift long-running tasks (e.g. network or database operations, complex calculations) from an app's main thread to a separate background thread. The most effective way to accomplish this task is at a class level. You can use AsyncTask class or IntentService class to organize background work. Once you have implemented an IntentService, it starts when needed and handles requests (Intents) using a worker thread.
When using IntentService, you should consider the following limitations:
- This class does not put results to UI, so to show results to users use Activity.
- Only one request is processed at a time.
- Any request processing can not be interrupted.
Tip 2. How to avoid ANR and stay responsive
The same approach of off-loading long-running operations from the UI thread will save your users from the "Application Not Responding" (ANR) dialog. What you need to do is to create a background worker thread by extending AsyncTask and implementing doInBackground() method.
Another option is to create a Thread or HandlerThread class of your own. Keep in mind that unless you specify "background" priority for the thread, it will slow down the app since the default thread priority is the same as of the UI thread.
Tip 3. How to initiate queries on separate threads
Displaying data is not immediate, although you can fasten it by using CursorLoader objects, which allows not to distract Activity from interacting with a user while query is processing in the background.
Armed with this object your app would initiate a separate background thread for each ContentProvider query and return results to Activity from which the query was called only when the query is finished.
Tip 4. What else you can do
- Use StrictMode to detect potentially lengthy operations you do in the UI thread.
- Use special tools, i.g. Systrace, Traceview, to find bottlenecks in your app responsiveness.
- Show progress to users with a progress bar.
- Display splash screens if initial setup is time-consuming.
Device battery life optimization
We cannot blame users for angrily uninstalling applications that abuse battery life. The main threats to battery life are:
- Regular wake-ups for updates
- Data transfer via EDGE and 3G
- Textual data parsing, regex without JIT
Tip 5. How to optimize networking issues
- Make your app skip operations if there is no connection; update only if 3G or WiFi is connected and there is no roaming.
- Choose compact data format, e.g. binary formats that combine text and binary data into one request.
- Use efficient parser; consider choosing stream parsers over tree parsers.
- For faster UX lessen round-trip times to server.
- When possible use framework GZIP libs for text data to make the best use of CPU resources.
Tip 6. How to optimize apps working in foreground
- When designing wakelocks, set the lowest level possible.
- To avoid battery costs caused by potential bugs you might have missed, use specific timeouts.
- Enable android:keepScreenOn.
- In addition to GC (garbage collection) consider recycling Java objects manually, e.g. XmlPullParserFactory and BitmapFactory; Matcher.reset(newString) for regex; StringBuilder.setLength(0).
- Mind synchronization issues, although it can be safe when driven by UI thread.
- Recycling strategies are used heavily in ListView.
- Use coarse network location not GPS when possible. Just compare 1mAh for GPS (25 sec. * 140mA) and 0.1mAh for network (2 seconds * 180mA).
- Make sure to unregister as GPS location updates can continue even after onPause(). When all applications unregister, users can enable GPS in Settings without blowing the battery budget.
- Since the calculation of a floating point requires lots of battery power, you might consider using microdegrees for bulk geo math and caching values when performing DPI tasks with DisplayMetrics.
Tip 7. How to optimize apps working in background
- Since each process requires 2MB and might be restarted when foreground apps need memory, make sure the services are short-lived.
- Keep memory usage low.
- Design app to update every 30 minutes but only if device is already awake.
- Services that pall or sleep are bad, that is why you should use AlarmManager or <receiver> manifest elements: stopSelf() when finished. When you start service using AlarmManager, apply the *_WAKEUP flags with caution. Let Android bin your application update together with the rest through setInexactRepeating(). When using <receiver>, enable/disable its components in manifest dynamically, especially when no-ops.
Tip 8. What else you can do
- Check current states of battery and network before launching a full update; wait for better states for bulk transfers.
- Provide users with battery usage options, e.g. update intervals and background behavior.
Implementing UI that leaves minimum memory footprints
Tip 9. How to identify layout performance problems
When creating UI sticking solely to basic features of layout managers, you risk to create memory abusing apps with annoying delays in the UI. The first step to implementation of a smooth, memory caring UI is to search your application for potential layout performance bottlenecks with Hierarchy Viewer tool included into Android SDK: <sdk>/tools/.
Another great tool for discovering performance issues is Lint. It scans application sources for possible bugs along with view hierarchy optimizations.
Tip 10. How to fix them
If layout performance results reveal certain drawbacks, you might consider to flatten the layout by converting it from LinearLayout class to RelativeLayout class, lowing level hierarchy.
To perfection and beyond
Even though each tip mentioned above might seem like a rather small improvement, you might see unexpectedly efficient results if these tips become an essential part of your daily coding. Let Google Play see more brilliant apps that work smoothly, quickly, and consume less battery power, bringing the Android world one more step closer to perfection.
By Anna Orlova on May 14, 2013
Article From: http://blog.azoft.com/android-application-development-tips/
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
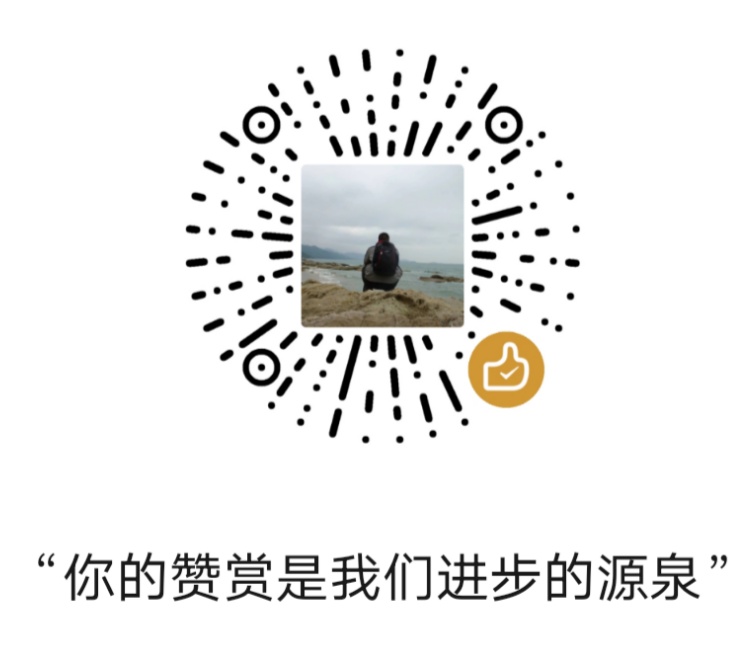
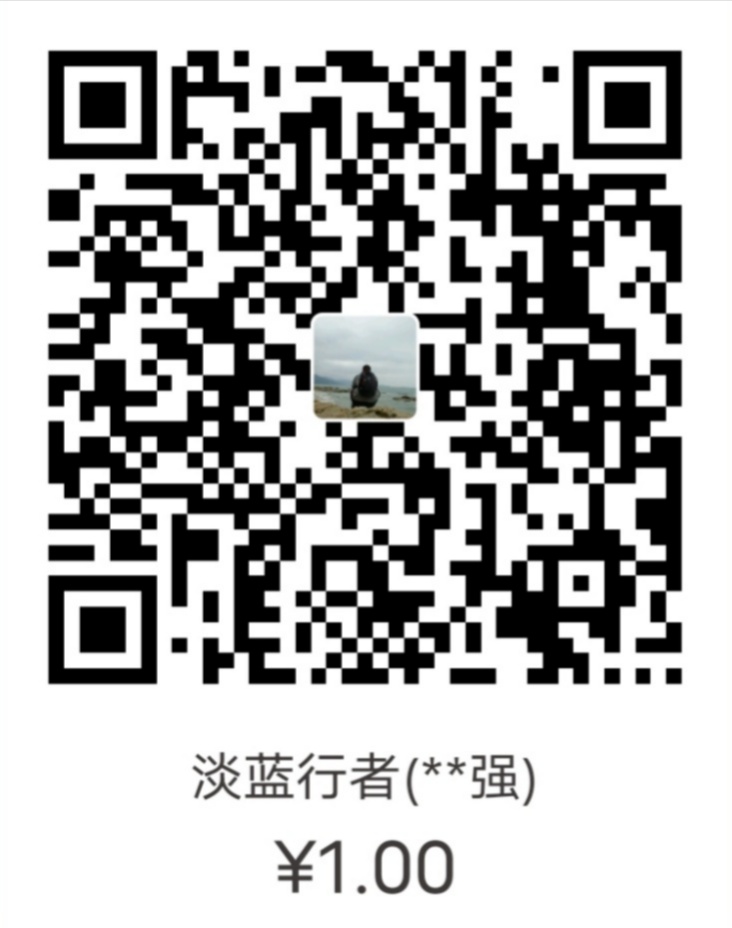