Angular2 HTTP 模块
在 Angular4 之前的 Angular2 中,HTTP API 方法的传参数形式如下:
http.get(url: string, options?: RequestOptionsArgs): Observable<Response>
http.post(url: string, body: any, options?: RequestOptionsArgs): Observable<Response>
- 1
- 2
- body 表示传递到服务器端的数据信息
- options 表示头部验证信息。
两个方法都返回一个可观察对象 Observable,我们可以通过 subscribe 方法得到里面的值并作后继处理。
this.http.post(url: string, body: any, options?: RequestOptionsArgs).subscribe(function (data) {
console.log(data)
})
- 1
- 2
- 3
RxJS 库
我们的服务可以返回 HTTP 响应对象 Response。但这可不是一个好主意! 数据服务的重点在于,对消费者隐藏与服务器交互的细节,我们最关心的还是获取到的返回数据信息,这时候我们就可以利用RxJS库来对事件流进行一些额外的处理。
RxJS(“Reactive Extensions” 的缩写)是一个被 Angular 认可的第三方库,它实现了异步可观察对象 (asynchronous observable) 模式。RxJS 库中包含很多对事件流进行处理的方法,例如map,distinctUntilChanged 等操作符。
针对返回数据是json格式的响应对象,可以把 Response 解析成 JavaScript 对象——只要调一下 response.json() 就可以了,这时候我们就可以利用map操作符来进行处理,例如,我们将上面的方法升级下
this.http.post(url: string, body: any, options?: RequestOptionsArgs).map((rsp: Response)=>{
return rsp.json()
}).subscribe((data) => {
console.log(data);
});
- 1
- 2
- 3
- 4
- 5
注意,这里 .map
用到了 RxJS 库,需要导入这个库。
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
- 1
- 2
Observer(观察者)是一个包含三个方法的对象
- next - 当 Observable 发送新值的时候,next 方法会被调用
- error - 当 Observable 内发生错误时,error 方法会被调用
- complete - 当 Observable 数据终止后,complete 方法会被调用,在调用 complete 方法之后,next 方法就不会再次被调用
Observable 对象转化为 Promise对象
虽然 Angular 的 HTTP Client API 返回的是 Observable<Response>
类型的对象,但我们也可以把它转成 Promise<Response>
。这很容易,只需要调用可观察对象 Observable< Response >
的方法 toPromise()
就能够进行转化。
this.http.post(url: string, body: any, options?: RequestOptionsArgs)
.toPromise()
.then((rsp: Response) => {
console.log(rsp)
});
- 1
- 2
- 3
- 4
- 5
使用 toPromise()
方法时要引入:
import 'rxjs/add/operator/toPromise';
- 1
Observable VS Promise
Observable | Promise |
---|---|
随着时间的推移发出多个值 | 返回单个值 |
可取消 | 不可取消 |
支持 map、filter、reduce 等操作符 | |
延迟执行,当订阅的时候才会开始执行 |
- - - - - - Angular2 与 Angular4 分界线 - - - - - -
Angular4 HTTP 模块
导入新的 HTTP Module
import { HttpClientModule } from '@angular/common/http';
- 1
特性一 默认 JSON 解析
需要注意的是,现在 JSON 是默认的数据格式,我们不需要再进行显式的解析
http.get(url).map(res => res.json()).subscribe(...)
- 1
现在我们可以这样写
http.get(url).subscribe(...)
- 1
特性二 支持拦截器
拦截器允许我们将中间件逻辑插入管线中
请求拦截器 (Request Interceptor)
import {
HttpRequest,
HttpHandler,
HttpEvent
} from '@angular/common/http';
@Injectable()
class JWTInterceptor implements HttpInterceptor {
constructor(private userService: UserService) {}
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const JWT = `Bearer ${this.userService.getToken()}`;
req = req.clone({
setHeaders: {
Authorization: JWT
}
});
return next.handle(req);
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
如果我们想要注册新的拦截器 (interceptor),我们需要实现 HttpInterceptor
接口,然后实现该接口中的 intercept
方法。
export interface HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>>;
}
- 1
- 2
- 3
需要注意的是,请求对象和响应对象必须是不可修改的 (immutable)。因此,我们在返回请求对象前,我们需要克隆原始的请求对象。
next.handle(req)
方法使用新的请求对象,调用底层的 XHR 对象,并返回响应事件流。
响应拦截器 (Response Interceptor)
@Injectable()
class JWTInterceptor implements HttpInterceptor {
constructor(private router: Router) {}
intercept(req: HttpRequest < any > ,
next: HttpHandler): Observable < HttpEvent < any >> {
return next.handle(req).map(event => {
if (event instanceof HttpResponse) {
if (event.status === 401) {
// JWT expired, go to login
}
}
return event;
}
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
响应拦截器可以通过在 next.handle(req)
返回的流对象 (即 Observable 对象) 上应用附加的 Rx 操作符来转换响应事件流对象。
接下来要应用 JWTInterceptor
响应拦截器的最后一件事是注册该拦截器,即使用 HTTP_INTERCEPTORS
作为 token,注册 multi Provider:
[{ provide: HTTP_INTERCEPTORS, useClass: JWTInterceptor, multi: true }]
- 1
特性三 进度事件
进度事件可以用于跟踪文件上传和下载
import {
HttpEventType,
HttpClient,
HttpRequest
} from '@angular/common/http';
http.request(new HttpRequest(
'POST',
URL,
body,
{
reportProgress: true
})).subscribe(event => {
if (event.type === HttpEventType.DownloadProgress) {
// {
// loaded:11, // Number of bytes uploaded or downloaded.
// total :11 // Total number of bytes to upload or download
// }
}
if (event.type === HttpEventType.UploadProgress) {
// {
// loaded:11, // Number of bytes uploaded or downloaded.
// total :11 // Total number of bytes to upload or download
// }
}
if (event.type === HttpEventType.Response) {
console.log(event.body);
}
})
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
如果我们想要跟踪文件上传或下载的进度,在创建请求对象时,我们需要配置 {reportProgress: true}
参数。
此外在回调函数中,我们通过 event.type
来判断不同的事件类型,从进行相应的事件处理。
HttpEventType
枚举定义如下:
export enum HttpEventType {
/**
* 表示请求已经被发送
*/
Sent,
/**
* 已接收到上传进度事件
*/
UploadProgress,
/**
* 已接收到响应状态码和响应头
*/
ResponseHeader,
/**
* 已接收到下载进度事件
*/
DownloadProgress,
/**
* 已接收全部响应,包含响应体
*/
Response,
/**
* 用户自定义事件,来自拦截器或后端
*/
User,
}
作者:abloume
来源:https://blog.csdn.net/u013451157/article/details/79519719
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
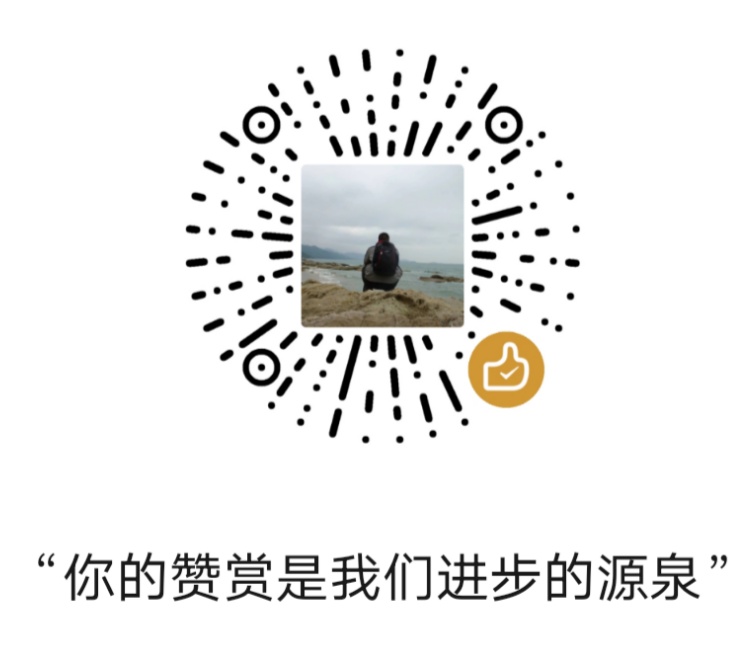
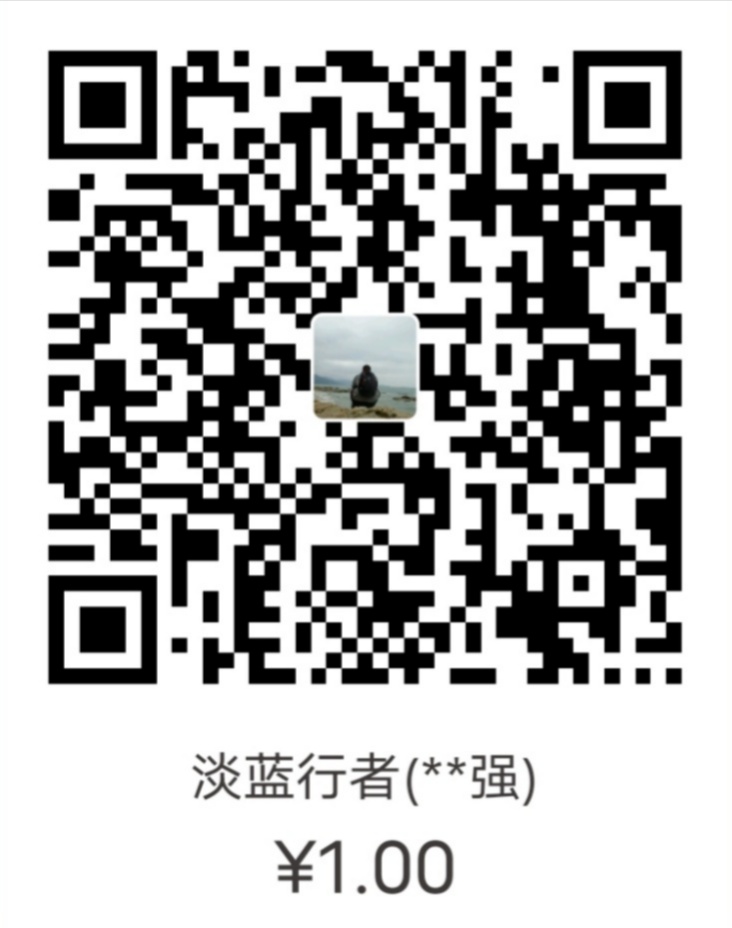