1. 背景
public static String read(String path){ //从给定位置获取文件 File file = new File(path); } CID 1565229(1 的 1 数):操控文件系统路径、文件名或 URI。 (PATH_MANIPULATION)5. sink: 使用被污染的值 path 构造路径或 URI。这可能允许攻击者访问、修改或测试关键或敏感文件的存在。 路径操作漏洞可以通过适当的输入验证进行解决。将允许不安全路径遍历的字符列入黑名单字符集可以改进输入的安全性,但推荐的方法是将预期字符集列入白名单。这里应该排除绝对路径和向上目录遍历。
2.解决方案
2.1 方案1
1 public class CleanPathUtil { 2 public static String cleanString(String str) { 3 if (str == null) return null; 4 StringBuilder sb = new StringBuilder(); 5 for (int i = 0; i < str.length(); ++i) { 6 sb.append(cleanChar(str.charAt(i))); 7 } 8 return sb.toString(); 9 } 10 11 private static char cleanChar(char ch) { 12 13 // 0 - 9 14 for (int i = 48; i < 58; ++i) { 15 if (ch == i) return (char) i; 16 } 17 18 // 'A' - 'Z' 19 for (int i = 65; i < 91; ++i) { 20 if (ch == i) return (char) i; 21 } 22 23 // 'a' - 'z' 24 for (int i = 97; i < 123; ++i) { 25 if (ch == i) return (char) i; 26 } 27 28 // other valid characters 29 switch (ch) { 30 case '/': 31 return '/'; 32 case '.': 33 return '.'; 34 case '-': 35 return '-'; 36 case '_': 37 return '_'; 38 case ',': 39 return ','; 40 case ' ': 41 return ' '; 42 case '!': 43 return '!'; 44 case '@': 45 return '@'; 46 case '#': 47 return '#'; 48 case '$': 49 return '$'; 50 case '%': 51 return '%'; 52 case '^': 53 return '^'; 54 case '&': 55 return '&'; 56 case '*': 57 return '*'; 58 case '(': 59 return '('; 60 case ')': 61 return ')'; 62 case '+': 63 return '+'; 64 case '=': 65 return '='; 66 case ':': 67 return ':'; 68 case ';': 69 return ';'; 70 case '?': 71 return '?'; 72 case '"': 73 return '"'; 74 case '<': 75 return '<'; 76 case '>': 77 return '>'; 78 case '`': 79 return '`'; 80 } 81 if (isChineseChar(ch)) 82 return ch; 83 log.error("[ALARM] Unrecognized character, Please check the path of input file name"); 84 return '%'; 85 } 86 87 // 根据Unicode编码判断中文汉字和符号 88 private static boolean isChineseChar(char c) { 89 Character.UnicodeBlock ub = Character.UnicodeBlock.of(c); 90 return ub == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS || ub == Character.UnicodeBlock.CJK_COMPATIBILITY_IDEOGRAPHS 91 || ub == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS_EXTENSION_A || ub == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS_EXTENSION_B 92 || ub == Character.UnicodeBlock.CJK_SYMBOLS_AND_PUNCTUATION || ub == Character.UnicodeBlock.HALFWIDTH_AND_FULLWIDTH_FORMS 93 || ub == Character.UnicodeBlock.GENERAL_PUNCTUATION; 94 } 95 96 }
2.2 方案2
1 public class StringVerifyUtil { 2 public static String verifyNonNullString(String input) { 3 char[] originalChars = input.toCharArray(); 4 char[] chars = new char[originalChars.length]; 5 for (int i = 0; i < originalChars.length; i++) { 6 chars[i] = purifyChar(originalChars[i]); 7 } 8 return new String(chars); 9 } 10 11 private static char purifyChar(char inputc) { 12 return inputc; 13 } 14 }
3 使用方法
1 public static String read(String path){ 2 //从给定位置获取文件 3 String cleanPath = StringVerifyUtil.verifyNonNullString(path); 4 File file = new File(cleanPath); 5 }
作者:屠城校尉杜
来源:https://www.cnblogs.com/dukedu/p/12522553.html
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
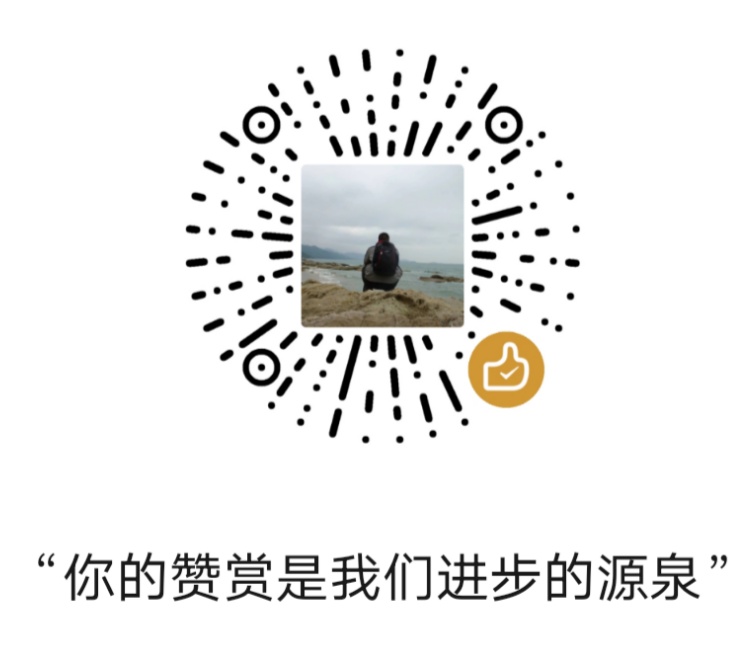
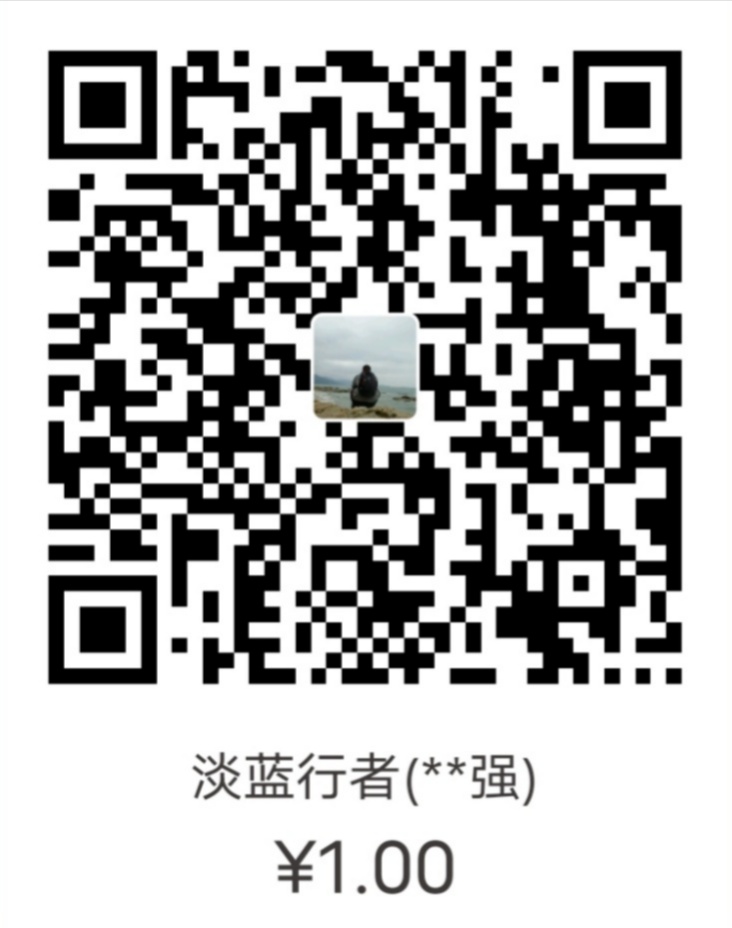