客户端:
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
import java.net.UnknownHostException;
import java.io.IOException;
public class UdpClient {
public static void main(String arg[]) {
String outstr;
if(arg.length >= 1)
outstr = arg[0];
else
outstr = "count";
try {
DatagramSocket socket = new DatagramSocket();
byte outblock[] = outstr.getBytes();
InetAddress address = InetAddress.getLocalHost();
DatagramPacket outpacket =
new DatagramPacket(outblock,outblock.length,address,8765);
socket.send(outpacket);
System.out.println("Client sent: " + outstr);
byte inblock[] = new byte[256];
DatagramPacket inpacket =
new DatagramPacket(inblock,inblock.length);
socket.receive(inpacket);
String instr = new String(inpacket.getData(),0,inpacket.getLength());
System.out.println("Client got: " + instr);
socket.close();
} catch(SocketException e) {
System.out.println(e);
} catch(UnknownHostException e) {
System.out.println(e);
} catch(IOException e) {
System.out.println(e);
}
}
}
服务器端:
import java.net.DatagramSocket;
import java.net.DatagramPacket;
import java.net.InetAddress;
import java.io.IOException;
import java.util.Date;
public class UdpServer {
public static void main(String arg[]) {
DatagramSocket socket = null;
int count = 0;
try {
socket = new DatagramSocket(8765);
} catch(IOException e) {
System.out.println(e);
}
while(true) {
try {
byte block[] = new byte[256];
DatagramPacket inpacket
= new DatagramPacket(block,block.length);
socket.receive(inpacket);
int length = inpacket.getLength();
System.out.println("Length of the data received: " + length);
byte inblock[] = inpacket.getData();
String inmsg = new String(inblock,0,length);
System.out.println("Server got: " + inmsg);
count++;
String outmsg;
if(inmsg.equals("date")) {
Date date = new Date();
outmsg = date.toString();
} else if(inmsg.equals("halt")) {
socket.close();
return;
} else if(inmsg.equals("count")) {
outmsg = "Number of messages: " + count;
} else {
outmsg = "What is " + inmsg + "?";
}
byte outblock[] = outmsg.getBytes();
InetAddress returnaddress = inpacket.getAddress();
int returnport = inpacket.getPort();
DatagramPacket outpacket = new DatagramPacket(
outblock,outblock.length,returnaddress,returnport);
socket.send(outpacket);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
(文/Offt)
本文来源:http://hi.baidu.com/offt/blog/item/41c4ed61e577d2780d33fa58.html
如果给你带来帮助,欢迎微信或支付宝扫一扫,赞一下。
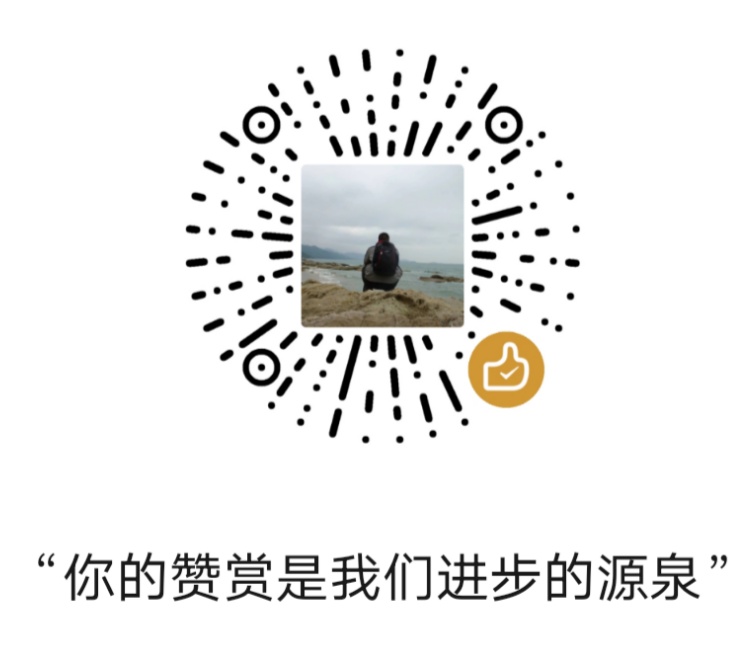
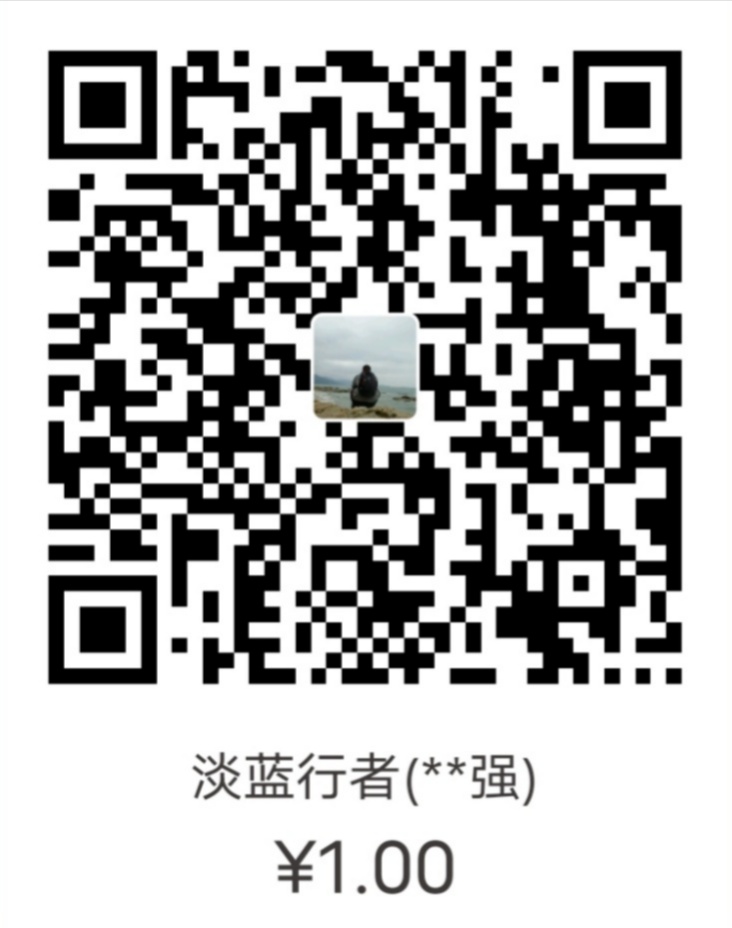